Fund user wallets
Funding methods
In order for users to fully participate in a game economy, such as by purchasing items, paying for transactions or trading with other users, they need to have the game's native currency, or other game-accepted currencies, in the wallets they have connected to the game. We have developed easy to use widgets that are designed to facilitate this process.
Widget | Use case |
---|---|
Bridge | The Bridge widget is ideal for users who have funds on L1 (e.g. Ethereum) and are looking to move some of the funds to zkEVM. 💡Example An item is sold on zkEVM for 0.2 USDC and I have 3 USDC in my L1 wallet that I'd love to use for purchasing the item. I can use the Bridge widget to move my L1 USDC tokens to L2 and complete the purchase. |
Swap | The Swap widget is ideal for users who have funds on zkEVM and are looking to swap tokens (e.g. USDC for IMX). 💡Example An item is sold on zkEVM for 0.2 USDC and I already have 4 ETH in my zkEVM wallet that I'd love to use for purchasing the item. I can use the Swap widget to swap my ETH tokens for USDC tokens and complete the purchase. |
On‑ramp | The On-ramp widget is idea for users who want to funds their zkEVM wallets using fiat (e.g. EUR). 💡Example An item is sold on zkEVM for 0.2 USDC and I do not have any funds on zkEVM on L1 (e.g. Ethereum). I can use the On-ramp widget to purchase USDC tokens using my Credit Card/Apple Pay/Google Pay and complete the purchase. |
Setup
The widgets are designed to be used client-side only and can be installed via CDN or package manager.
- npm module
- JavaScript via CDN
Prerequisites
Node Version 20 or later
To install nvm
follow these instructions. Once installed, run:
nvm install --lts
- (Optional) To enable Code Splitting (importing only the SDK modules you need) there are additional prerequisites.
Install the Immutable SDK
Run the following command in your project root directory.
- npm
- yarn
npm install -D @imtbl/sdk
# if necessary, install dependencies
npm install -D typescript ts-node
yarn add --dev @imtbl/sdk
# if necessary, install dependencies
yarn add --dev typescript ts-node
The Immutable SDK is still in early development. If experiencing complications, use the following commands to ensure the most recent release of the SDK is correctly installed:
- npm
- yarn
rm -Rf node_modules
npm cache clean --force
npm i
rm -Rf node_modules
yarn cache clean
yarn install
To add the SDK to an application using the CDN the following script can be placed inside the head tag.
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
Bridge
Bridges facilitate communication between blockchains through the transfer of information and assets. The sample code code below gives a starting point for integrating the widget into your application.
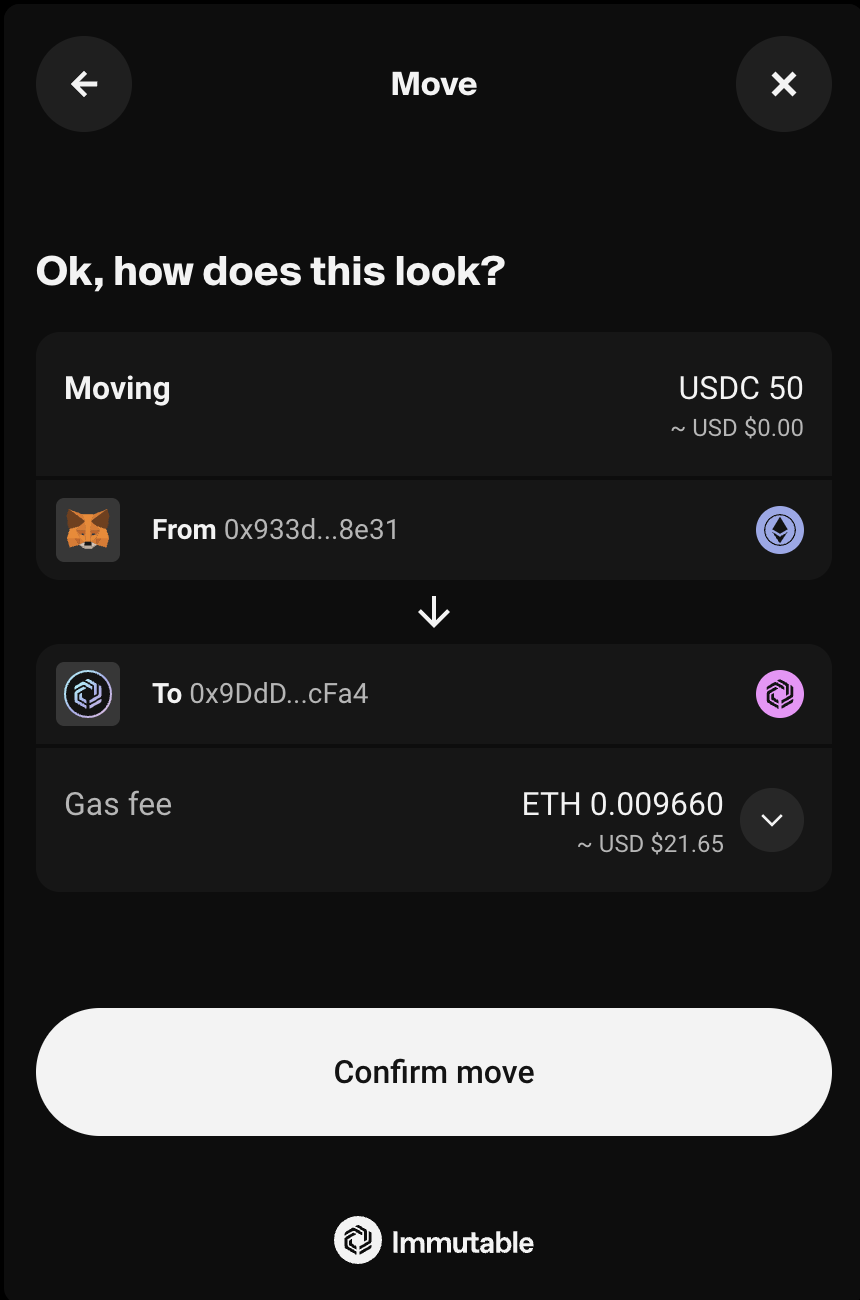
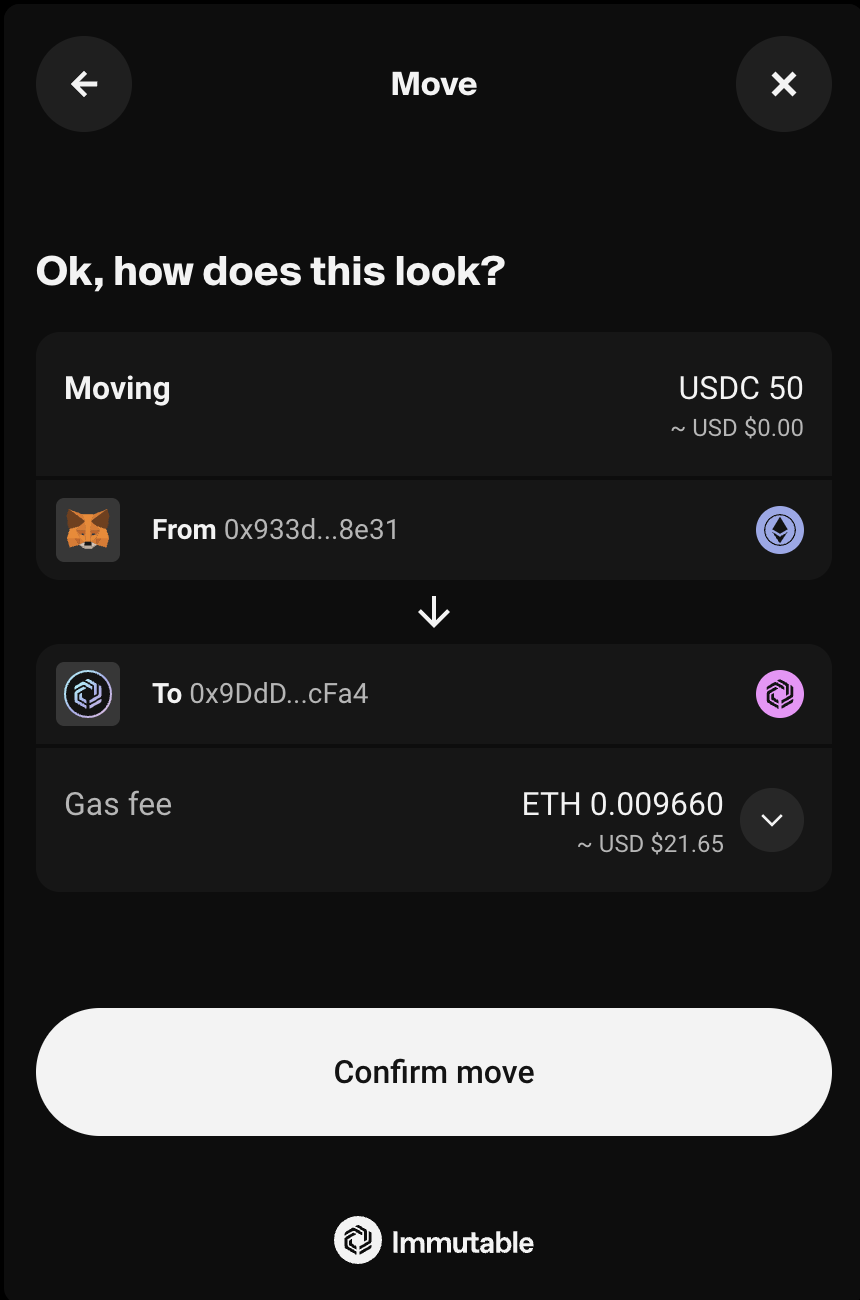
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// create Checkout SDK
// Initialise widgets, create bridge widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const bridge = widgets.create(checkout.WidgetType.BRIDGE);
bridge.mount('bridge');
})();
}, []);
return <div id="bridge" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="bridge"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const bridge = widgets.create(ImmutableCheckout.WidgetType.BRIDGE);
bridge.mount('bridge');
})();
</script>
</body>
</html>
To learn more about this widget explore the Bridge widget documentation.
Swap
A token swap refers to exchanging one cryptocurrency token directly for another without using fiat money (e.g. USD). The sample code code below gives a starting point for integrating the widget into your application.
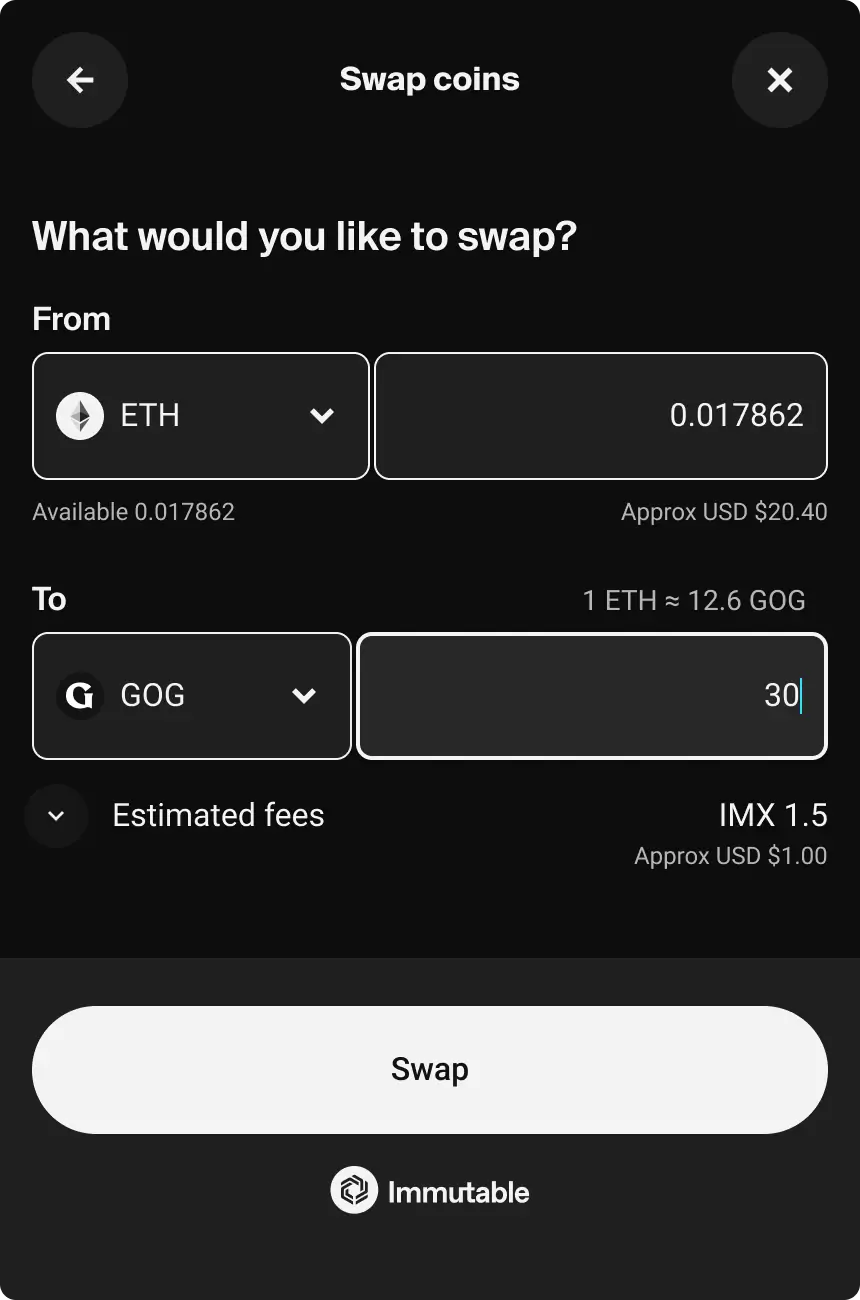
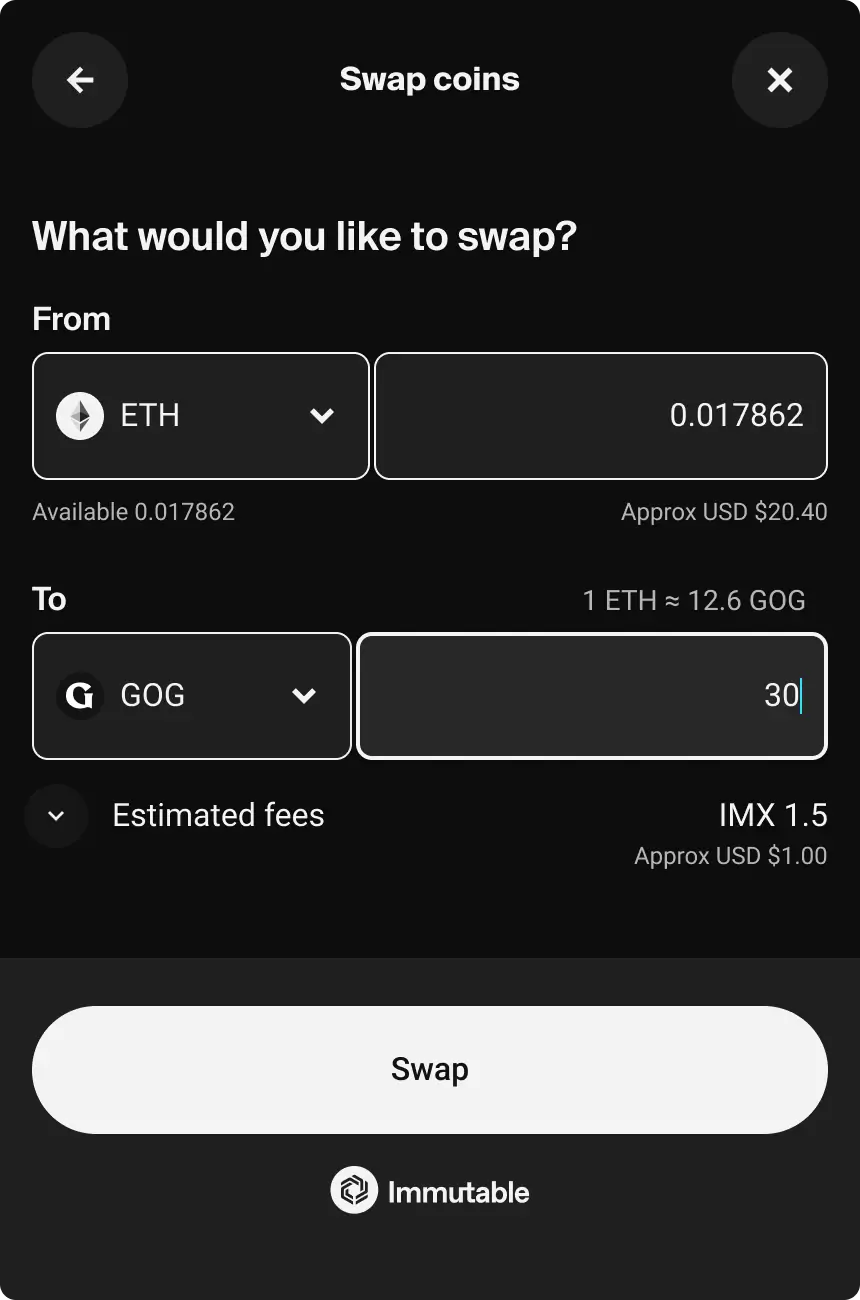
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise widgets, create swap widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const swap = widgets.create(checkout.WidgetType.SWAP)
swap.mount("swap");
})();
}, []);
return (<div id="swap" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="swap"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const swap = widgets.create(ImmutableCheckout.WidgetType.SWAP);
swap.mount('swap');
})();
</script>
</body>
</html>
To learn more about this widget explore the Swap Widget documentation.
On-ramp
On-ramping tokens refers to exchanging fiat currency (e.g. USD) directly to buy cryptocurrency. The sample code code below gives a starting point for integrating the widget into your application.
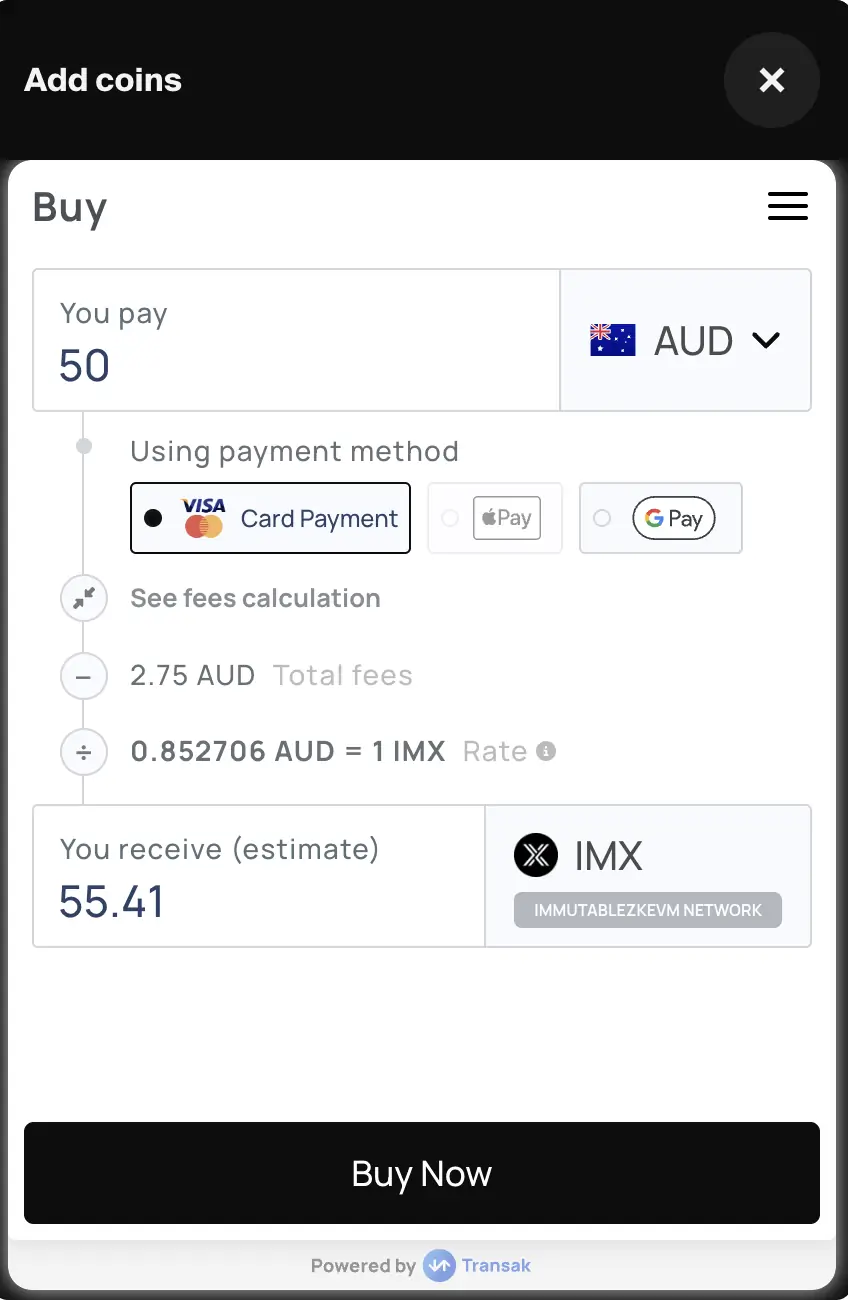
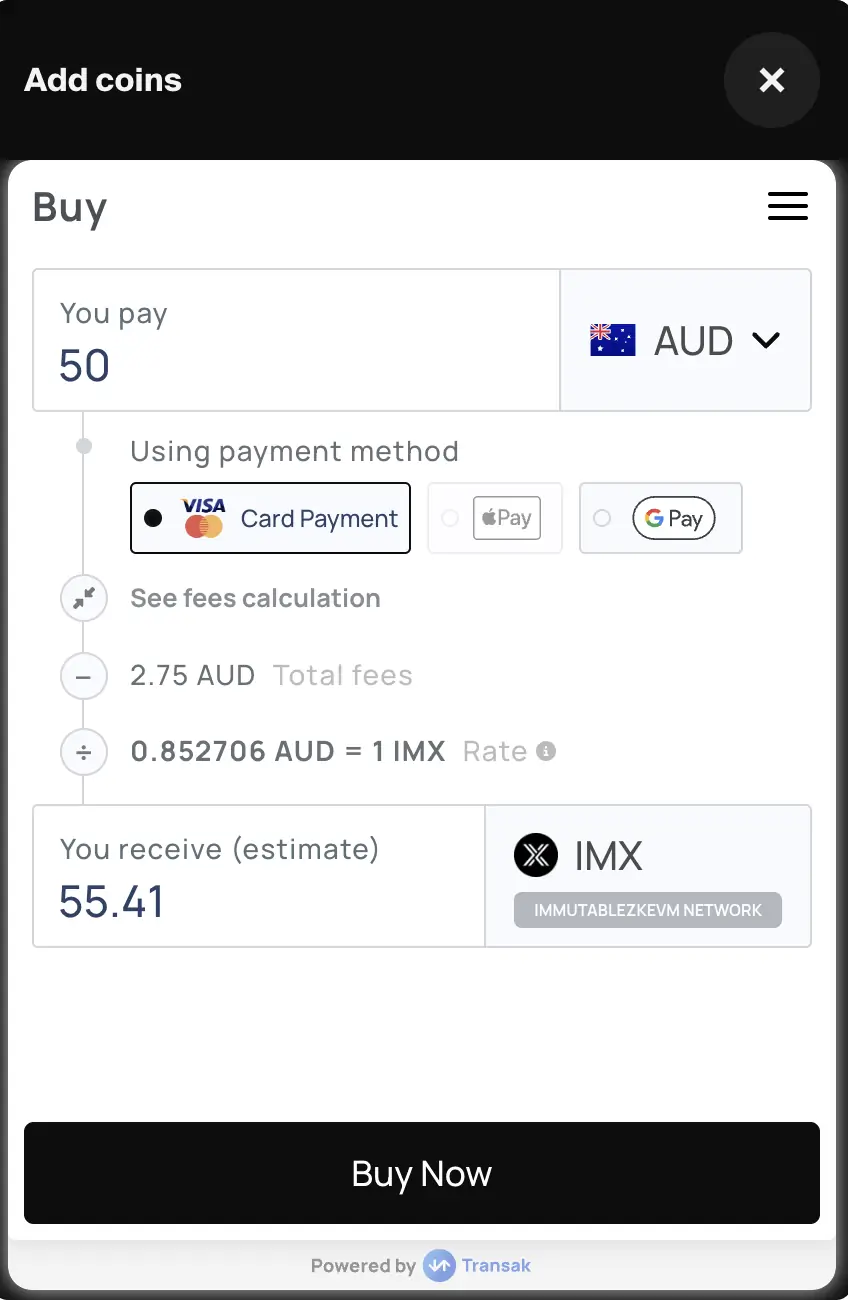
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise widgets, create onramp widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const onramp = widgets.create(checkout.WidgetType.ONRAMP)
onramp.mount("onramp");
})();
}, [checkout]);
return (<div id="onramp" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="onramp"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const onramp = widgets.create(ImmutableCheckout.WidgetType.ONRAMP);
onramp.mount('onramp');
})();
</script>
</body>
</html>
To learn more about this widget explore the On-ramp Widget documentation.