On-ramp tokens
The On-ramp widget is a drop-in solution for web-based games and marketplaces that simplifies the process of purchasing tokens with fiat (e.g. USD) onto the Immutable zkEVM network.
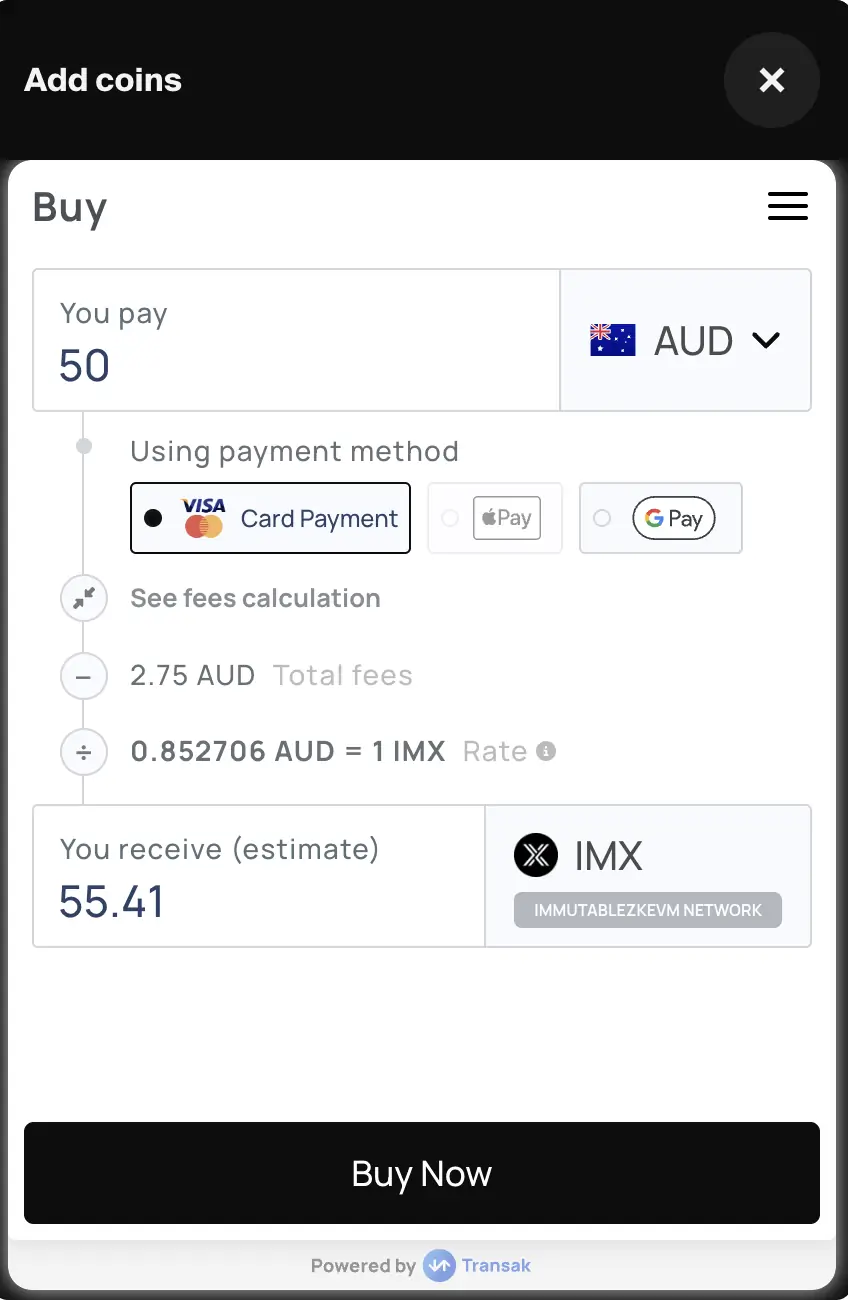
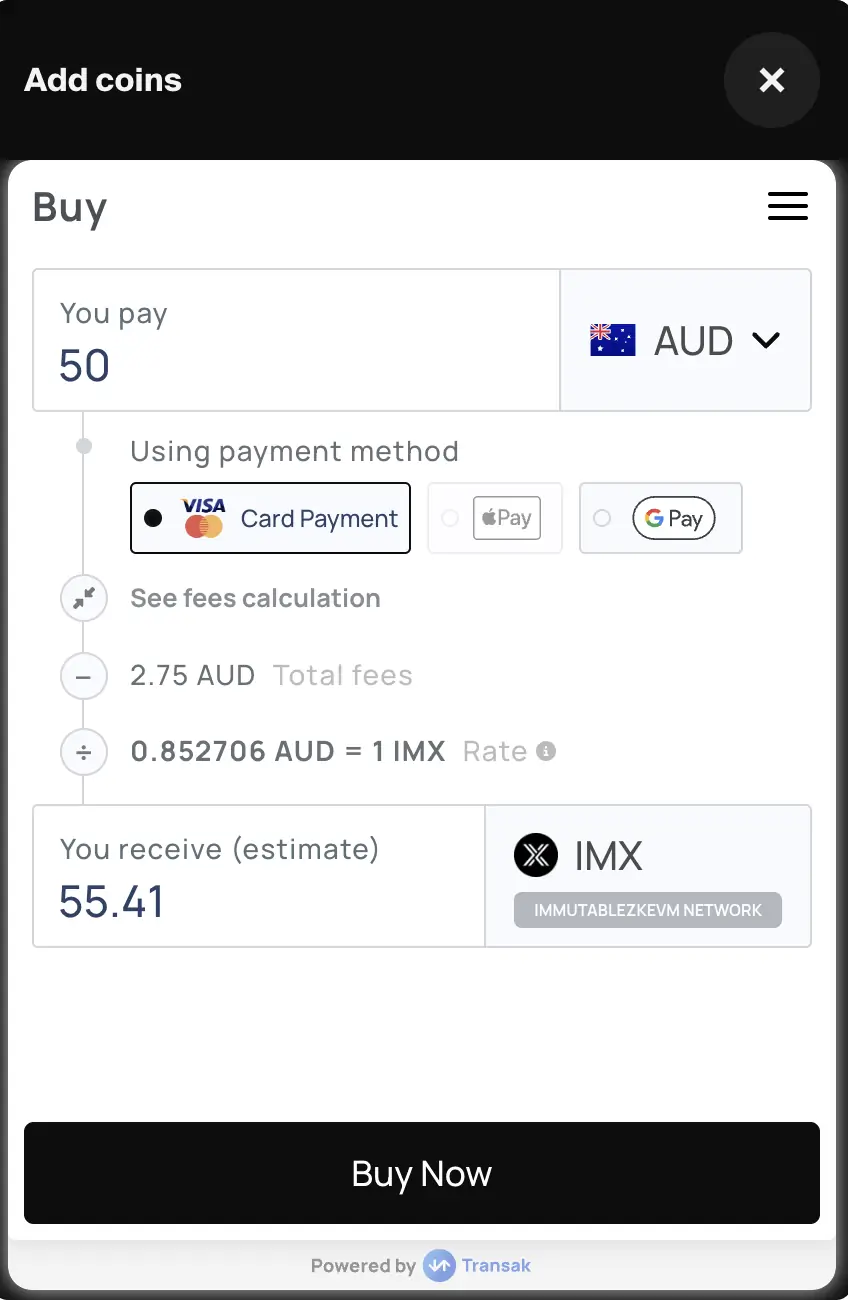
Getting started
Once you have completed the widget setup, use the WidgetsFactory to create an on-ramp widget.
In order to mount the widget, call the mount()
function and pass in the id
attribute of the target element you wish to mount it to.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise widgets, create onramp widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const onramp = widgets.create(checkout.WidgetType.ONRAMP)
onramp.mount("onramp");
})();
}, [checkout]);
return (<div id="onramp" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="onramp"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const onramp = widgets.create(ImmutableCheckout.WidgetType.ONRAMP);
onramp.mount('onramp');
})();
</script>
</body>
</html>
Parameters
The mount()
function can also take in parameters to be passed into the widget.
Parameters are treated as transient and will be reset after the widget is unmounted.
Parameter | Description |
---|---|
tokenAddress | The address of the ERC20 contract to buy. |
amount | The ERC20 token amount to buy. |
walletProviderName | The wallet provider name to use for the onramp widget. |
- React
- JavaScript
import { checkout } from "@imtbl/sdk";
// @ts-ignore
const onramp = widgets.create(checkout.WidgetType.ONRAMP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// add parameters for the onramp widget
onramp.mount('onramp', { tokenAddress: '0xaddress', amount: '10' })
// When mounting the widget, pass in the parameters to use
onramp.mount('onramp', {contractAddress: '0xaddress', amount: '10', walletProviderName:'Metamask'})
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme will create the widget with that theme. If this is not passed the configuration will be set by default.
- React
- JavaScript
import { checkout } from "@imtbl/sdk";
//@ts-ignore add configuration for the onramp widget
const onramp = widgets.create(checkout.WidgetType.ONRAMP, { config: { theme: checkout.WidgetTheme.DARK }})
// You can update the onramp widget's configuration by calling the update() method
onramp.update({config: {theme: checkout.WidgetTheme.LIGHT}})
// When creating the widget, pass in the configuration
const onramp = widgets.create(ImmutableCheckout.WidgetType.ONRAMP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// Update the widget config by calling update()
onramp.update({config: { theme: ImmutableCheckout.WidgetTheme.LIGHT }});
Events
Onramp widget events are emitted when critical actions have been taken by the user or key states have been reached. Below is a table of the possible events for the Onramp Widget.
Event Type | Description | Event Payload |
---|---|---|
OnRampEventType.SUCCESS | A user successfully on-ramped tokens to their wallet. | OnRampSuccess |
OnRampEventType.FAILURE | On-ramping of tokens failed. | OnRampFailed |
OnRampEventType.CLOSE_WIDGET | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. |
- React
- JavaScript
You can use the addListener()
function to tap into the events and provider handlers. Use the removeListener()
function to stop listening to that event.
import { checkout } from "@imtbl/sdk";
// @ts-ignore
const onramp = widgets.create(checkout.WidgetType.ONRAMP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// add event listeners for the onramp widget
onramp.addListener(checkout.OnRampEventType.SUCCESS, (data: checkout.OnRampSuccess) => {
console.log("success", data);
});
onramp.addListener(checkout.OnRampEventType.FAILURE, (data: checkout.OnRampFailed) => {
console.log("failure", data);
});
onramp.addListener(checkout.OnRampEventType.CLOSE_WIDGET, () => {
onramp.unmount();
});
// remove event listeners for the onramp widget
onramp.removeListener(checkout.OnRampEventType.SUCCESS);
onramp.removeListener(checkout.OnRampEventType.FAILURE);
onramp.removeListener(checkout.OnRampEventType.CLOSE_WIDGET);
const onramp = widgets.create(ImmutableCheckout.WidgetType.ONRAMP);
// add event listeners for the onramp widget
onramp.addListener(ImmutableCheckout.OnRampEventType.SUCCESS, (data) => {
console.log('success', data);
});
onramp.addListener(ImmutableCheckout.OnRampEventType.FAILURE, (data) => {
console.log('failure', data);
});
onramp.addListener(ImmutableCheckout.OnRampEventType.CLOSE_WIDGET, (data) => {
console.log('close', data);
});
// remove event listeners for the onramp widget
onramp.removeListener(ImmutableCheckout.OnRampEventType.SUCCESS);
onramp.removeListener(ImmutableCheckout.OnRampEventType.FAILURE);
onramp.removeListener(ImmutableCheckout.OnRampEventType.CLOSE_WIDGET);
Sample code
This sample code gives you a good starting point for integrating the onramp widget into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [onramp, setOnramp] =
useState<checkout.Widget<typeof checkout.WidgetType.ONRAMP>>();
// Initialise widgets, create onramp widget
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const onramp = widgets.create(checkout.WidgetType.ONRAMP, { config: { theme: checkout.WidgetTheme.DARK }})
setOnramp(onramp)
})();
}, [checkout]);
// mount onramp widget and add event listeners
useEffect(() => {
if(!onramp) return;
onramp.mount("onramp");
onramp.addListener(checkout.OnRampEventType.SUCCESS, (data: checkout.OnRampSuccess) => {
console.log("success", data);
});
onramp.addListener(checkout.OnRampEventType.FAILURE, (data: checkout.OnRampFailed) => {
console.log("failure", data);
});
onramp.addListener(checkout.OnRampEventType.CLOSE_WIDGET, () => {
onramp.unmount();
});
}, [onramp])
return (<div id="onramp" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="onramp"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const onramp = widgets.create(ImmutableCheckout.WidgetType.ONRAMP, { config: { theme: ImmutableCheckout.WidgetTheme.DARK }});
onramp.mount('onramp');
onramp.addListener(ImmutableCheckout.OnRampEventType.SUCCESS, (data) => {
console.log('success', data);
});
onramp.addListener(ImmutableCheckout.OnRampEventType.FAILURE, (data) => {
console.log('failure', data);
});
onramp.addListener(ImmutableCheckout.OnRampEventType.CLOSE_WIDGET, () => {
onramp.unmount();
});
})();
</script>
</body>
</html>