Swap tokens
The Swap widget is a drop-in solution for web-based games and marketplaces that simplifies the process of swapping tokens.
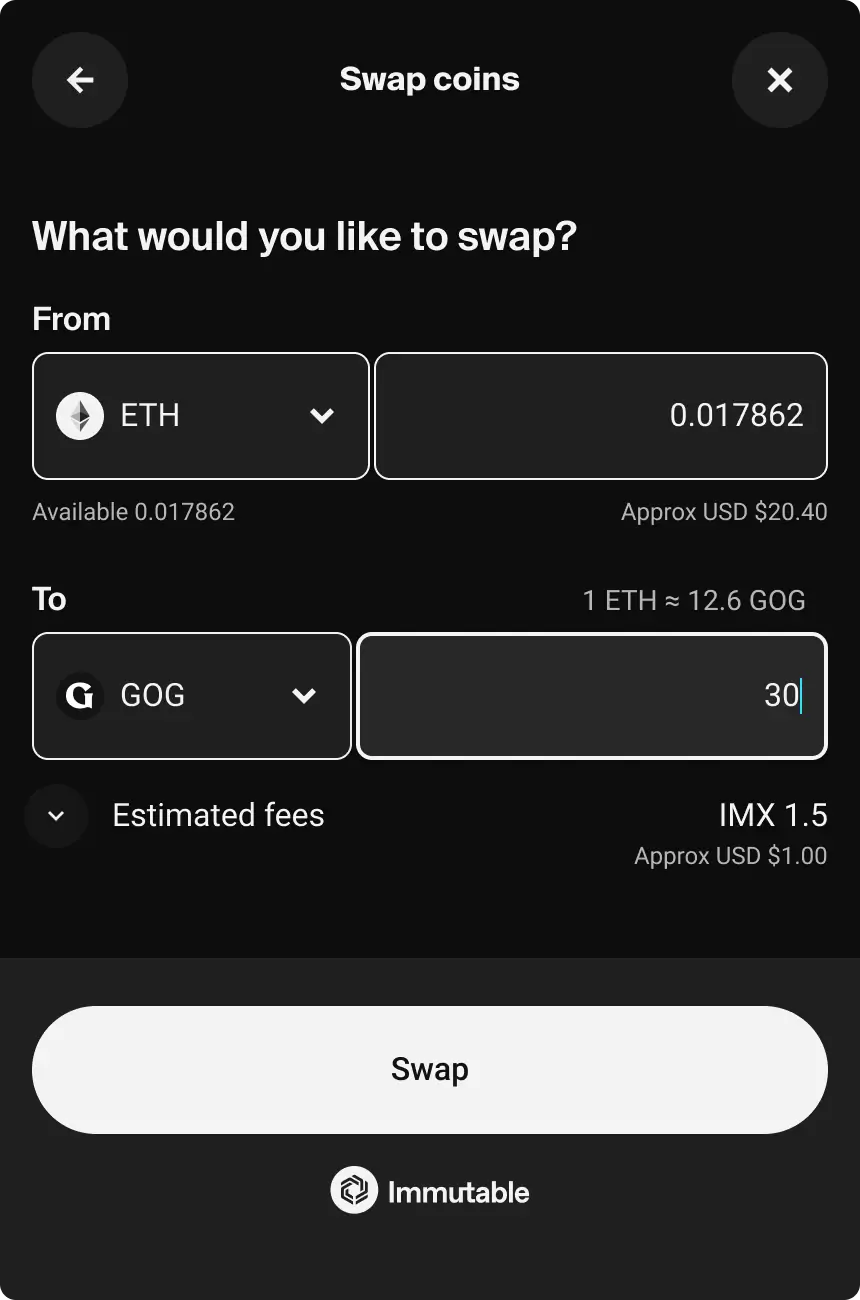
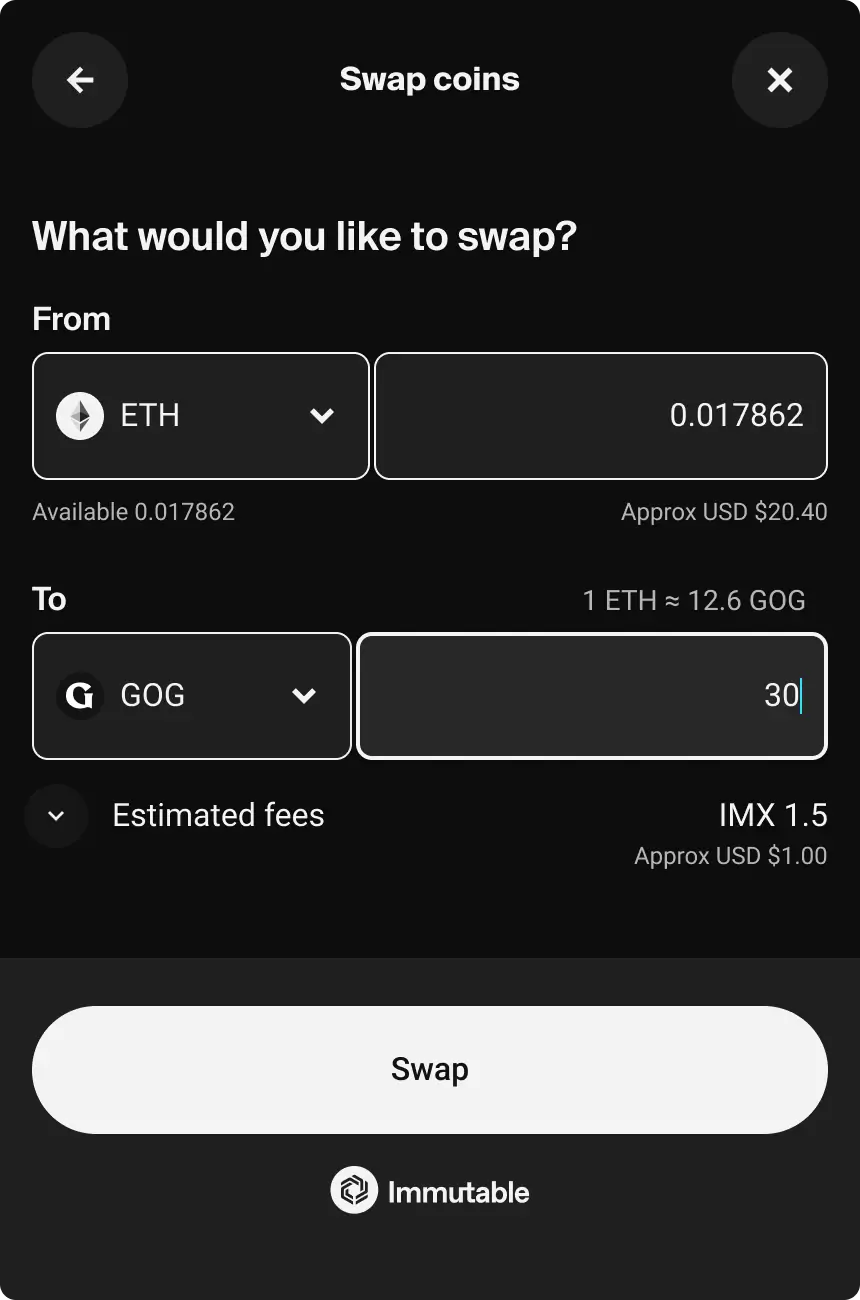
Getting started
Once you have completed the widget setup, use the WidgetsFactory to create a connect widget.
In order to mount the widget, call the mount()
function and pass in the id
attribute of the target element you wish to mount it to.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise widgets, create swap widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const swap = widgets.create(checkout.WidgetType.SWAP)
swap.mount("swap");
})();
}, []);
return (<div id="swap" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="swap"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const swap = widgets.create(ImmutableCheckout.WidgetType.SWAP);
swap.mount('swap');
})();
</script>
</body>
</html>
Parameters
The mount()
function can also take in parameters to be passed into the widget.
Parameters are treated as transient and will be reset after the widget is unmounted.
Property | Description |
---|---|
fromTokenAddress | The address of the ERC20 contract swapping from. Use NATIVE to set the swapping from token to IMX . This will prefill the token field in the form. |
toTokenAddress | The address of the ERC20 contract swapping to. Use NATIVE to set the swapping to token to IMX . This will prefill the token field in the form. |
amount | The ERC20 token amount to swap. |
walletProviderName | The wallet provider name to use for the swap widget. |
- React
- JavaScript
import { checkout } from "@imtbl/sdk";
// @ts-ignore
const swap = widgets.create(checkout.WidgetType.SWAP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// When calling the mount function, pass in the parameters to use
swap.mount('swap', { fromTokenAddress: '0x123', toTokenAddress: '0x999', amount: '10' })
// When calling the mount function, pass in the parameters to use
swap.mount("swap", { fromTokenAddress: '0x123', amount: '10' });
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme will create the widget with that theme. If this is not passed the configuration will be set by default.
Configuration will persist after the widget is unmounted. You can always update a widget's configuration later by calling the update()
method.
Property | Description |
---|---|
SwapWidgetConfiguration | The configuration type to be used with the Swap Widget. |
- React
- JavaScript
import { checkout } from "@imtbl/sdk";
//@ts-ignore When creating the widget, pass in the configuration
const swap = widgets.create(checkout.WidgetType.SWAP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// Update the widget config by calling update()
swap.update({config: {theme: checkout.WidgetTheme.LIGHT}});
// When creating the widget, pass in the configuration
const bridge = widgets.create(ImmutableCheckout.WidgetType.BRIDGE,
{ config: { theme: ImmutableCheckout.WidgetTheme.DARK }}
);
// Update the widget config by calling update()
bridge.update({ config: { theme: ImmutableCheckout.WidgetTheme.LIGHT }});
For more information on the configurations across all the Checkout Widgets (e.g. theme) review the Configuration section in our Setup page.
Events
Swap widget events are emitted when critical actions have been taken by the user or key states have been reached. Below is a table of the possible events for the Swap Widget.
Event Type | Description | Event Payload |
---|---|---|
SwapEventType.SUCCESS | A user successfully connected their wallet. | SwapSuccess |
SwapEventType.FAILURE | There was a problem when swapping tokens. | SwapFailed |
SwapEventType.REJECTED | The swap transaction was rejected. | SwapRejected |
SwapEventType.CLOSE_WIDGET | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. |
- React
- JavaScript
All the widget events are emitted with type IMTBL_SWAP_WIDGET_EVENT
.
Access the event types via the key (e.g. checkoutWidgets.SwapEventType.SUCCESS
, checkoutWidgets.SwapEventType.CLOSE_WIDGET
).
import { checkout } from "@imtbl/sdk";
// @ts-ignore
const swap = widgets.create(checkout.WidgetType.SWAP,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// add event listeners for the swap widget
swap.addListener(checkout.SwapEventType.SUCCESS, (data: checkout.SwapSuccess) => {
console.log("success", data);
});
swap.addListener(checkout.SwapEventType.FAILURE, (data: checkout.SwapFailed) => {
console.log("failure", data);
});
swap.addListener(checkout.SwapEventType.REJECTED, (data: checkout.SwapRejected) => {
console.log("failure", data);
});
swap.addListener(checkout.SwapEventType.CLOSE_WIDGET, () => {
swap.unmount();
});
// remove event listeners for the swap widget
swap.removeListener(checkout.SwapEventType.SUCCESS);
swap.removeListener(checkout.SwapEventType.FAILURE);
swap.removeListener(checkout.SwapEventType.REJECTED);
swap.removeListener(checkout.SwapEventType.CLOSE_WIDGET);
All the widget events are emitted as imtbl-swap-widget
.
Specify the event type by the string value (e.g. success
, close-widget
).
const swap = widgets.create(ImmutableCheckout.WidgetType.SWAP);
// add event listeners for the swap widget
swap.addListener(ImmutableCheckout.SwapEventType.SUCCESS, (data) => {
console.log('success', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.FAILURE, (data) => {
console.log('failure', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.REJECTED, (data) => {
console.log('rejected', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.CLOSE_WIDGET, (data) => {
console.log('close', data);
});
// remove event listeners for the swap widget
swap.removeListener(ImmutableCheckout.SwapEventType.SUCCESS);
swap.removeListener(ImmutableCheckout.SwapEventType.FAILURE);
swap.removeListener(ImmutableCheckout.SwapEventType.REJECTED);
swap.removeListener(ImmutableCheckout.SwapEventType.CLOSE_WIDGET);
Sample code
This sample code gives you a good starting point for integrating the connect widget into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [swap, setSwap] =
useState<checkout.Widget<typeof checkout.WidgetType.SWAP>>();
// Initialise widgets, create swap widget
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const swap = widgets.create(checkout.WidgetType.SWAP, { config: { theme: checkout.WidgetTheme.DARK }})
setSwap(swap)
})();
}, []);
// mount swap widget and add event listeners
useEffect(() => {
if(!swap) return;
swap.mount("swap");
swap.addListener(checkout.SwapEventType.SUCCESS, (data: checkout.SwapSuccess) => {
console.log("success", data);
});
swap.addListener(checkout.SwapEventType.FAILURE, (data: checkout.SwapFailed) => {
console.log("failure", data);
});
swap.addListener(checkout.SwapEventType.REJECTED, (data: checkout.SwapRejected) => {
console.log("rejected", data);
});
swap.addListener(checkout.SwapEventType.CLOSE_WIDGET, () => {
swap.unmount();
});
}, [swap])
return (<div id="swap" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="swap"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const swap = widgets.create(ImmutableCheckout.WidgetType.SWAP, { config: { theme: ImmutableCheckout.WidgetTheme.DARK }});
swap.mount('swap');
swap.addListener(ImmutableCheckout.SwapEventType.SUCCESS, (data) => {
console.log('success', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.FAILURE, (data) => {
console.log('failure', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.REJECTED, (data) => {
console.log('rejected', data);
});
swap.addListener(ImmutableCheckout.SwapEventType.CLOSE_WIDGET, () => {
swap.unmount();
});
})();
</script>
</body>
</html>