Get NFT data
Immutable's Blockchain Data APIs allow developers to retrieve data about non-fungible (ERC721) and semi-fungible (ERC1155) NFTs on Immutable-supported chains.
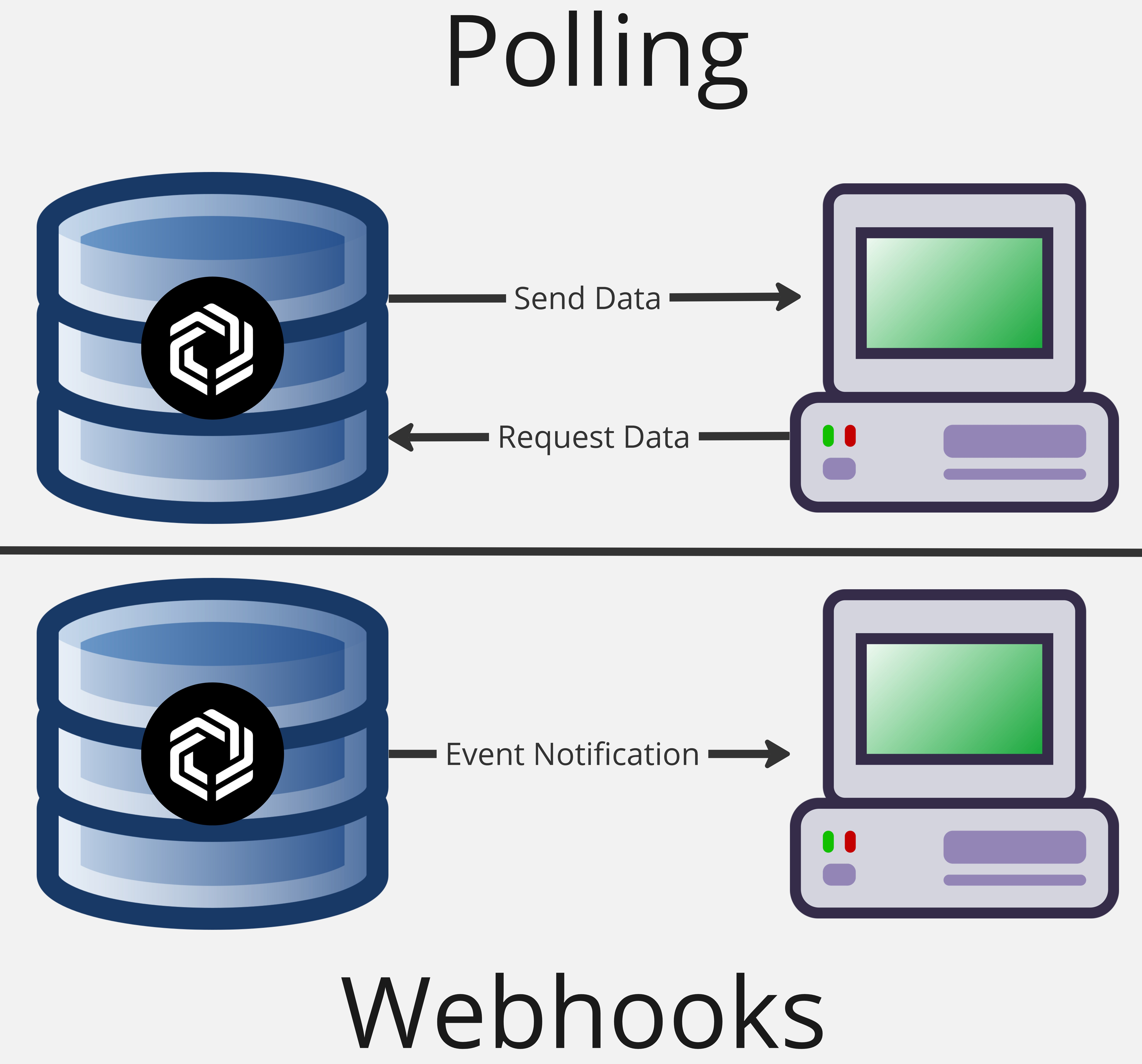
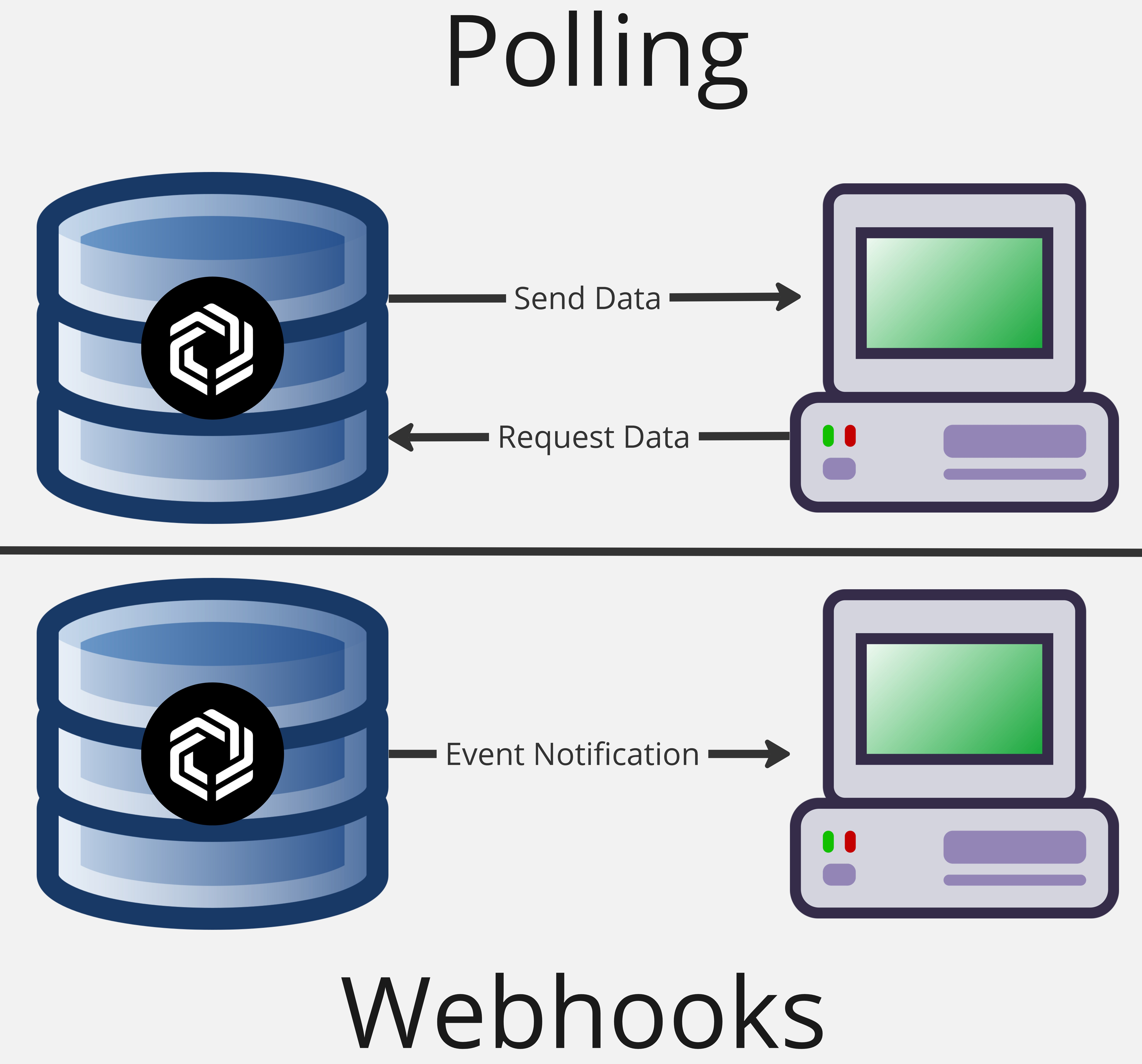
List all NFTs
This request returns a paginated list of all the NFTs that belong to a particular chain.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
fromUpdatedAt | Datetime to use as the oldest updated timestamp | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listAllNFTs(): Promise<blockchainData.Types.ListNFTsResult> {
return await client.listAllNFTs({
chainName: 'imtbl-zkevm-testnet',
});
}
GET {baseURL}/v1/chains/{chain_name}/nfts
Note: The NFT owner is not included in the response of this query. Use listNFTOwners
for this feature.
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"token_id": "1",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"contract_type": "ERC721",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"metadata_synced_at": "2022-08-16T17:43:26.991388Z",
"metadata_id": "ae83bc80-4dd5-11ee-be56-0242ac120002",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_link": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
],
"total_supply": "1"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
List NFTs from a particular collection
This request returns a paginated list of all the NFTs that belong to a particular collection.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | Yes |
tokenId | An array of token IDs strings to filter the returned list by | No |
fromUpdatedAt | Datetime to use as the oldest updated timestamp | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listNFTsByCollection(
contractAddress: string,
tokenId: string[],
): Promise<blockchainData.Types.ListNFTsResult> {
return await client.listNFTs({
chainName: 'imtbl-zkevm-testnet',
contractAddress,
tokenId,
});
}
GET {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts
Note: The NFT owner is not included in the response of this query. Use listNFTOwners
for this feature.
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"token_id": "1",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"contract_type": "ERC721",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"metadata_synced_at": "2022-08-16T17:43:26.991388Z",
"metadata_id": "ae83bc80-4dd5-11ee-be56-0242ac120002",
"name": "Sword",
"description": "2022-08-16T17:43:26.991388Z",
"image": "https://some-url",
"external_link": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
],
"total_supply": "1"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
List NFTs owned by a particular account address
This request returns a paginated list of all the NFTs that belong to a particular account address (owner).
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
accountAddress | The public address of a wallet holding NFTs | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | No |
fromUpdatedAt | Datetime to use as the oldest updated timestamp | No |
tokenId | The token ID string you want to return | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listNFTsByAccountAddress(
chainName: string,
contractAddress: string,
accountAddress: string,
): Promise<blockchainData.Types.ListNFTsResult> {
return await client.listNFTsByAccountAddress({
chainName,
contractAddress,
accountAddress,
});
};
GET {baseURL}/v1/chains/{chainName}/accounts/{address}/nfts
Note: The NFT owner is not included in the response of this query. Use listNFTOwners
for this feature.
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"token_id": "1",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"contract_type": "ERC721",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"metadata_synced_at": "2022-08-16T17:43:26.991388Z",
"metadata_id": "ae83bc80-4dd5-11ee-be56-0242ac120002",
"name": "Sword",
"description": "This is a super awesome sword",
"image": "https://some-url",
"external_link": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
],
"balance": "11"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
Note: The NFT owner is not included in the response of this query. Use listNFTOwners
for this feature.
Get the details of a single NFT
This request allows you to get details of a specific NFT, including details like the collection it belongs to, when it was minted, and its metadata.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
contractAddress | The contract address of ERC721 contract you want to filter by | Yes |
tokenId | The token ID you want to return | Yes |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
When an asset is minted, its metadata is cached by Immutable's blockchain data indexer, making it accessible via the the Blockchain Data APIs.
When an NFT's metadata is updated, requesting a "metadata refresh" updates the cached information to ensure that changes are available via the API.
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function getNFT(chainName: string, contractAddress: string, tokenId: string): Promise<blockchainData.Types.GetNFTResult> {
return await client.getNFT({
chainName: chainName,
contractAddress: contractAddress,
tokenId: tokenId,
});
}
GET {baseURL}/v1/chains/{chainName}/collections/{contractAddress}/nfts/{tokenId}
Note: The NFT owner is not included in the response of this query. Use listNFTOwners
for this feature.
Example response
{
"result": {
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"token_id": "1",
"contract_address": "0x8a90cab2b38dba80c64b7734e58ee1db38b8992e",
"contract_type": "ERC1155",
"indexed_at": "2022-08-16T17:43:26.991388Z",
"updated_at": "2022-08-16T17:43:26.991388Z",
"metadata_synced_at": "2022-08-16T17:43:26.991388Z",
"metadata_id": "ae83bc80-4dd5-11ee-be56-0242ac120002",
"name": "Sword",
"description": "This is a super awesome sword",
"image": "https://some-url",
"external_link": "https://some-url",
"animation_url": "https://some-url",
"youtube_url": "https://some-url",
"attributes": [
{
"trait_type": "Aqua Power",
"value": "Happy"
}
],
"total_supply": "100"
}
}
List all NFT owners
This request allows you to get the details of all owners.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
fromUpdatedAt | Datetime to use as the oldest updated timestamp | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listAllNFTOwners(): Promise<blockchainData.Types.ListNFTOwnersResult> {
return await client.listAllNFTOwners({
chainName: 'imtbl-zkevm-testnet',
});
}
GET {baseURL}/v1/chains/{chainName}/nft-owners
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"token_id": "1",
"account_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"quantity": "1",
"balance": "1",
"updated_at": "2022-08-16T17:43:26.991388Z"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
List NFT owners of particular token ID
This request allows you to get the details of all owners that have custody of a single NFT. For ERC721 NFTs, only a single owner will be returned. If multi-owner token standards are added to the Data APIs, this API may return a list in future.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
contractAddress | The address of the contract | Yes |
tokenId | The address of the contract | Yes |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listNFTOwnersByTokenId(
contractAddress: string,
tokenId: string,
): Promise<blockchainData.Types.ListNFTOwnersResult> {
return await client.listNFTOwners({
chainName: 'imtbl-zkevm-testnet',
contractAddress,
tokenId,
});
}
GET {baseURL}/v1/chains/{chainName}/collections/{contractAddress}/nfts/{tokenId}/owners
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"token_id": "1",
"account_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"quantity": "1",
"balance": "1",
"updated_at": "2022-08-16T17:43:26.991388Z"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}
List NFT owners from a particular collection
This request returns a paginated list of all the NFT owners that belong to a particular collection.
Request parameters
Parameter | Description | Required |
---|---|---|
chainName | String representing the name of the chain. A list of available chains can be found here. | Yes |
contractAddress | The address of the contract | Yes |
accountAddress | The public address of a wallet holding NFTs | No |
fromUpdatedAt | Datetime to use as the oldest updated timestamp | No |
Pagination parameters | Parameters such as page_size and page_cursor that allow you to control the size and order of the data retrieved | No |
- SDK
- API
import { blockchainData } from '@imtbl/sdk';
import { client } from '../lib';
export async function listNFTOwnersByContractAddress(
contractAddress: string,
): Promise<blockchainData.Types.ListNFTOwnersResult> {
return await client.listNFTOwnersByContractAddress({
chainName: 'imtbl-zkevm-testnet',
contractAddress,
});
}
GET {baseURL}/v1/chains/{chainName}/collections/{contractAddress}/owners
Example response
{
"result": [
{
"chain": {
"id": "eip155:13473",
"name": "imtbl-zkevm-testnet"
},
"contract_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"token_id": "1",
"account_address": "0x5a019874f4fae314b0eaa4606be746366e661306",
"quantity": "1",
"balance": "1",
"updated_at": "2022-08-16T17:43:26.991388Z"
}
],
"page": {
"previous_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0=",
"next_cursor": "ewogICJ0eXBlIjogInByZXYiLAogICJpdGVtIjogewogICAgImlkIjogNjI3NTEzMCwKICAgICJjcmVhdGVkX2F0IjogIjIwMjItMDktMTNUMTc6MDQ6MTIuMDI0MTI2WiIKICB9Cn0="
}
}