View balances
The Wallet widget is a drop-in solution for web-based games and marketplaces that simplifies the process of checking wallet balances on the Immutable zkEVM and Ethereum networks as well as accessing the bridging and swapping features.
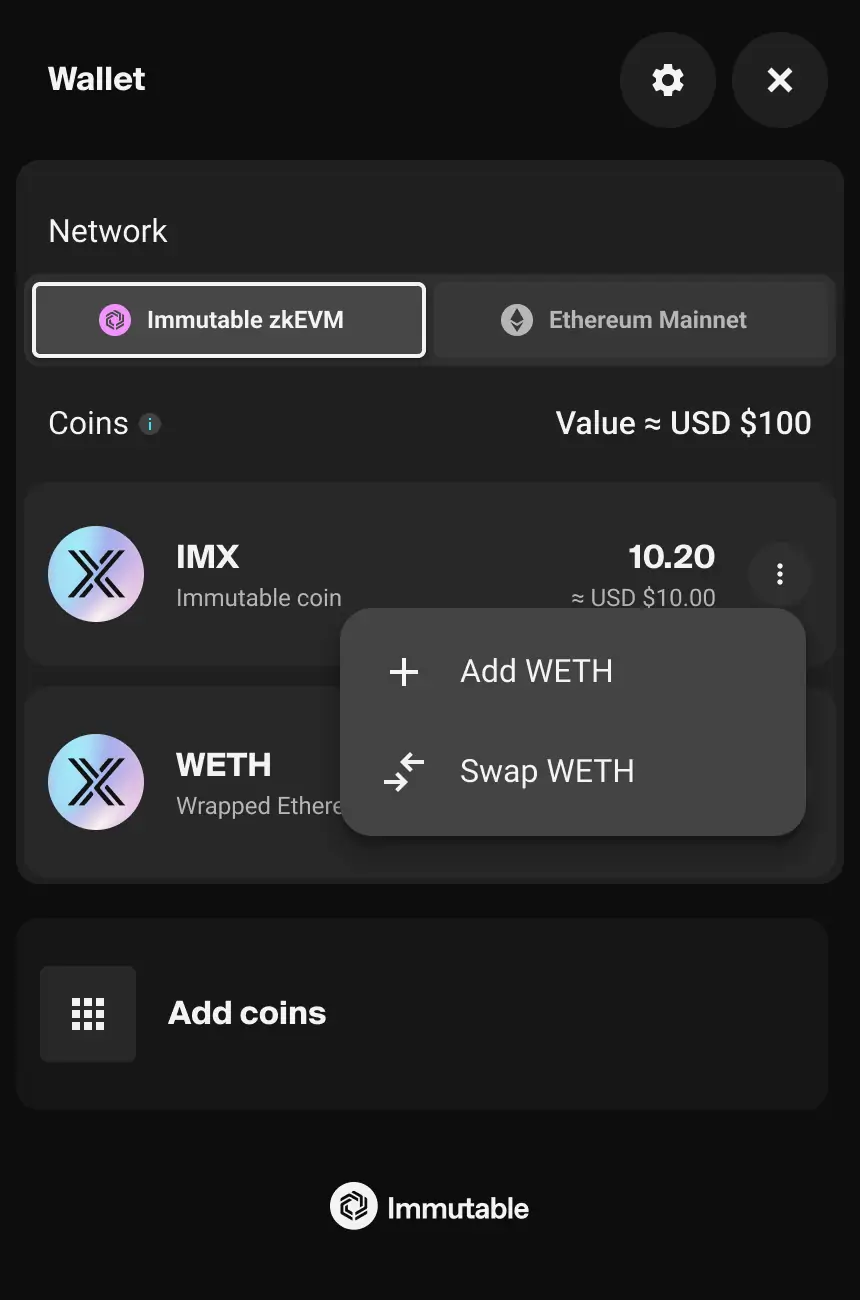
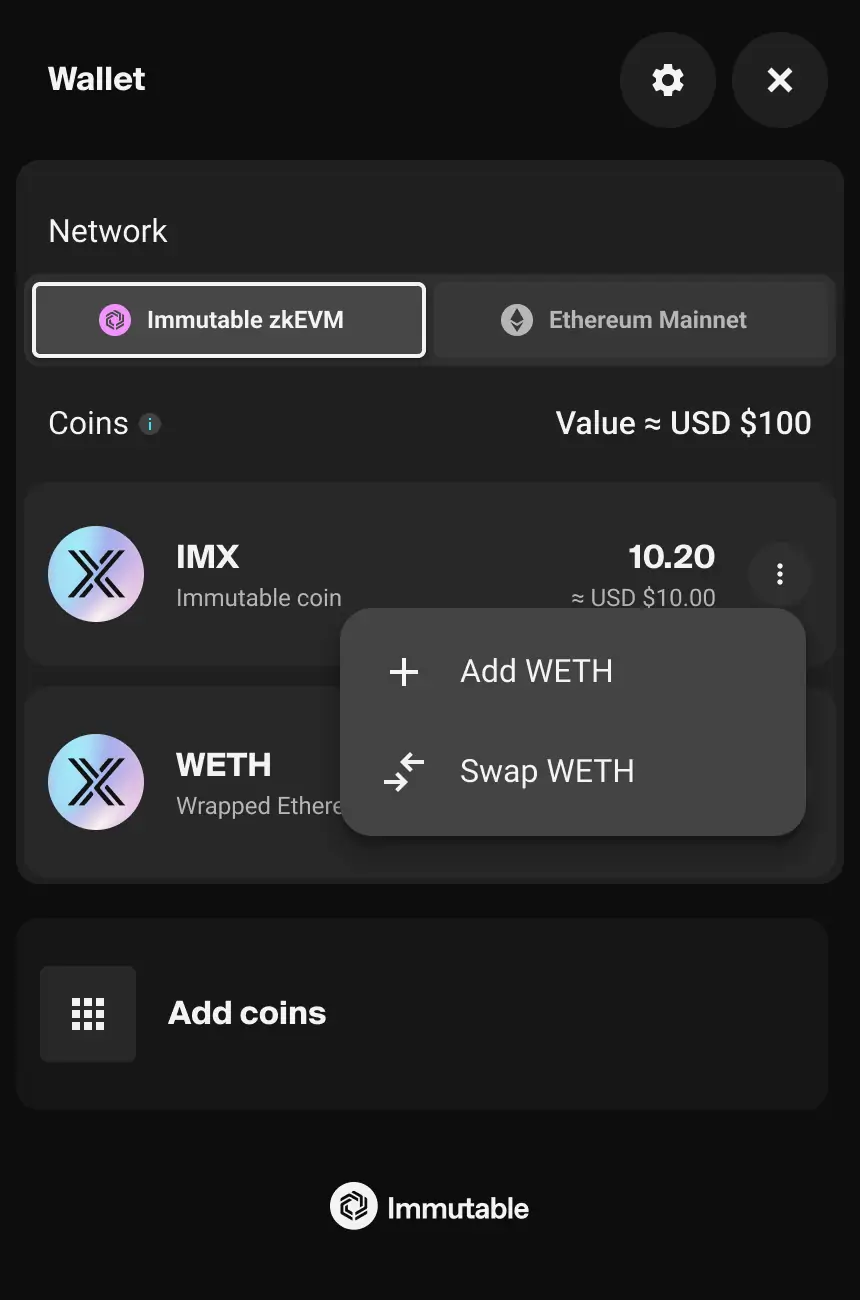
Getting started
Once you have completed the widget setup, use the WidgetsFactory to create a wallet widget.
In order to mount the widget, call the mount()
function and pass in the id
attribute of the target element you wish mount it to.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise widgets, create wallet widget and mount
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const wallet = widgets.create(checkout.WidgetType.WALLET)
wallet.mount("wallet");
})();
}, []);
return (<div id="wallet" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="wallet"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const wallet = widgets.create(ImmutableCheckout.WidgetType.WALLET);
wallet.mount('wallet');
})();
</script>
</body>
</html>
Parameters
The mount()
function can also take in parameters to be passed into the widget.
Parameters are treated as transient and will be reset after the widget is unmounted.
Parameter | Description |
---|---|
walletProviderName | The name of the wallet provider to use. |
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme will create the widget with that theme. If this is not passed the configuration will be set by default.
Configuration will persist after the widget is unmounted. You can always update a widget's configuration later by calling the update()
method.
Property | Description |
---|---|
WalletWidgetConfiguration | The configuration type to be used with the Wallet Widget. |
- React
- JavaScript
import { checkout } from "@imtbl/sdk";
// @ts-ignore When creating the widget, pass in the configuration
const wallet = widgets.create(checkout.WidgetType.WALLET, {
config: {
theme: checkout.WidgetTheme.DARK
},
walletConfig: {
showNetworkButton: true,
showDisconnectButton: true
}
});
// Update the widget config by calling update()
wallet.update({
config: {
theme: checkout.WidgetTheme.LIGHT
},
walletConfig: {
showNetworkButton: false,
showDisconnectButton: false
}
});
// When creating the widget, pass in the configuration
const wallet = widgets.create(
ImmutableCheckout.WidgetType.WALLET,
{ config: { theme: ImmutableCheckout.WidgetTheme.DARK }}
);
// Update the widget config by calling update()
wallet.update({
config: { theme: ImmutableCheckout.WidgetTheme.LIGHT }
});
For more information on the configurations across all the Checkout Widgets (e.g. theme) review the Configuration section in our Setup page.
Events
Wallet widget events are emitted when critical actions have been taken by the user or key states have been reached. Below is a table of the possible events for the Wallet Widget.
Event Type | Description | Event Payload |
---|---|---|
WalletEventType.NETWORK_SWITCH | A user switched their network from the wallet. | WalletNetworkSwitch |
WalletEventType.DISCONNECT_WALLET | The user disconnected their wallet. | WalletDisconnect |
WalletEventType.CLOSE_WIDGET | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. |
- React
- JavaScript
You can use the addListener()
function to tap into the events and provider handlers. Use the removeListener()
function to stop listening to that event.
import { checkout } from "@imtbl/sdk";
//@ts-ignore
const wallet = widgets.create(checkout.WidgetType.WALLET,
{ config: { theme: checkout.WidgetTheme.DARK }}
);
// add event listeners for the wallet widget
wallet.addListener(checkout.WalletEventType.NETWORK_SWITCH, (data: checkout.WalletNetworkSwitch) => {
console.log("network switch", data);
});
wallet.addListener(checkout.WalletEventType.DISCONNECT_WALLET, (data: checkout.WalletDisconnect) => {
console.log("wallet disconnected", data);
});
wallet.addListener(checkout.WalletEventType.CLOSE_WIDGET, () => {
wallet.unmount();
});
// remove event listeners for the wallet widget
wallet.removeListener(checkout.WalletEventType.NETWORK_SWITCH);
wallet.removeListener(checkout.WalletEventType.DISCONNECT_WALLET);
wallet.removeListener(checkout.WalletEventType.CLOSE_WIDGET);
You can use the addListener()
function to tap into the events and provider handlers. Use the removeListener()
function to stop listening to that event.
// add event listeners for the connect widget
connect.addListener(ImmutableCheckout.WalletEventType.NETWORK_SWITCH, (data) => {
console.log("network switch", data);
});
connect.addListener(ImmutableCheckout.WalletEventType.DISCONNECT_WALLET, (data) => {
console.log("wallet disconnected", data);
});
connect.addListener(ImmutableCheckout.WalletEventType.CLOSE_WIDGET, (data) => {
console.log('close', data);
});
// remove event listeners for the connect widget
connect.removeListener(ImmutableCheckout.WalletEventType.NETWORK_SWITCH);
connect.removeListener(ImmutableCheckout.WalletEventType.DISCONNECT_WALLET);
connect.removeListener(ImmutableCheckout.WalletEventType.CLOSE_WIDGET);
Sample code
This sample code gives you a good starting point for integrating the connect widget into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [wallet, setWallet] =
useState<checkout.Widget<typeof checkout.WidgetType.WALLET>>();
// Initialise widgets, create wallet widget
useEffect(() => {
(async () => {
const widgets = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK },
});
const wallet = widgets.create(checkout.WidgetType.WALLET, {config: {theme: checkout.WidgetTheme.DARK}})
setWallet(wallet)
})();
}, []);
// mount wallet widget and add event listeners
useEffect(() => {
if(!wallet) return;
wallet.mount("wallet");
wallet.addListener(checkout.WalletEventType.NETWORK_SWITCH, (data: checkout.WalletNetworkSwitch) => {
console.log("network switch", data);
});
wallet.addListener(checkout.WalletEventType.DISCONNECT_WALLET, (data: checkout.WalletDisconnect) => {
console.log("wallet disconnected", data);
});
wallet.addListener(checkout.WalletEventType.CLOSE_WIDGET, () => {
wallet.unmount();
});
}, [wallet])
return (<div id="wallet" />);
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="wallet"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const wallet = widgets.create(ImmutableCheckout.WidgetType.WALLET, {
config: { theme: ImmutableCheckout.WidgetTheme.DARK }
});
wallet.mount('wallet');
wallet.addListener(ImmutableCheckout.WalletEventType.NETWORK_SWITCH, (data) => {
console.log('network switch', data);
});
wallet.addListener(ImmutableCheckout.WalletEventType.DISCONNECT_WALLET, (data) => {
console.log('wallet disconnected', data);
});
wallet.addListener(ImmutableCheckout.WalletEventType.CLOSE_WIDGET, () => {
wallet.unmount();
});
})();
</script>
</body>
</html>