Minting API
On this page we show you how to use Immutable's Minting API. Our Minting API streamlines the minting process, enabling seamless creation of NFTs with just one API call. Whether you're minting one NFT or hundreds, our API makes it easy and efficient.
Th Minting API is now available for all game studios and users with Hub accounts. See rate limits for more details.
The Minting API is available to all developers using Immutable's ERC721 and ERC1155 preset contracts.
Benefits of Minting API?
Immutable's Minting API simplifies the process of minting NFTs at scale by providing a user-friendly API interface. This makes Immutable's platform more accessible as Immutable solves the following issues when minting at scale:
- Local nonce tracking and management
- Transaction lifecycle tracking
- Continuously sourcing IMX for minting
- Expedited metadata indexing by submitting metadata through APIs
- Token ID reconciliation for the
mintBatchByQuantity()
function
To ensure compatibility with the Minting API, game studios are required to use Immutable's preset contracts.
Using the Minting API
To access the Minting API, you must have a Hub account, as it requires your organization’s Secret API Key.
Send a POST request to the mint-requests
endpoint to initiate minting. The Minting API processes requests asynchronously and supports batch minting. Immutable optimizes these batches, meaning multiple mint requests may share the same transaction hash to reduce gas costs.
Metadata can be set during the minting process or added later via the Metadata Refresh API.
A reference_id
is required for each minting request and must be unique within a collection. It can be the same value as the token_id
if using the mintBatch()
function. If a mint attempt fails, the same reference_id
can be reused until successful. However, reusing a reference_id
from a successfully minted asset or using the same reference_id
for multiple assets within the same request will result in an error. This rule also applies to ERC1155 collections, even when the token_id
is repeated.
Regardless of the chosen method for metadata setup, content creators must ensure that their local metadata is synchronized with Immutable's Blockchain Data API. Marketplaces and other ecosystem partners may not always use the Blockchain Data API to reference an asset's metadata.
While the Minting API allows minters to set up asset metadata via a simple POST request, this feature does not remove the necessity for studios to host their own metadata.
For a comprehensive understanding of how an asset's metadata file, the Blockchain Data API, and the baseURI
field work together to index a collection's metadata, please refer to this guide.
Minting API rate limits
The following rate limits exist for the Minting API.
Testnet: All Game Studios and Users
Action | Rate Limit |
---|---|
# of Token IDs per request | 100 |
Total # of NFTs/SFTs requested per minute | 100 |
Mainnet: Game Studios with an Immutable Relationship
Action | Rate Limit |
---|---|
# of Token IDs per request | 100 |
Total # of NFTs/SFTs requested per minute | 2,000 |
Mainnet: Game Studios and Users
Action | Rate Limit |
---|---|
# of Token IDs per request | 100 |
Total # of NFTs/SFTs requested per minute | 100 |
See default rate limits for all API calls here.
The above rate limits reflect rate new mint requests are accepted by the Minting API, they do not represent the rate that assets are minted to the chain which varies due to a variety of factors.
Note that for ERC1155 collections the amount minted does not impact rate limits, NFT/SFT only refers to the number of different token_id
s included in the request.
Minting API prerequisites
If you are deploying a collection via Hub, checking the 'Enable Minting API' during deployment will automatically grant the minter role to the Immutable Minting API.
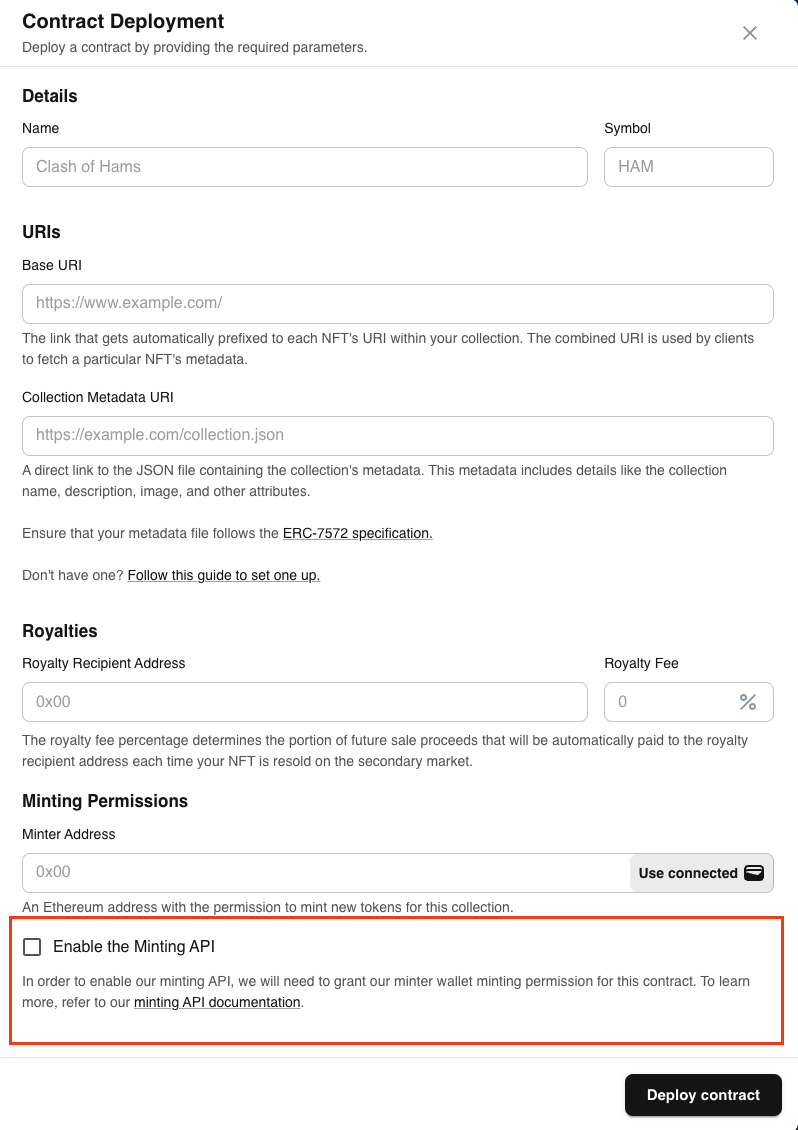
If you are not using the Immutable Hub to deploy your collection, you will need perform the following steps:
Create an account in Immutable Hub
Deploy a collection to testnet or mainnet using Immutable's preset contracts. This can be done via Hub or manual deployment. If you manually deploy your contract ensure you link the collection to your Immutable Hub account.
Get the
contractAddress
of the deployed contractGet a
secretAPIKey
from Immutable Hub. This will be required in the header of the API call. If using the SDK please check out this guide as a reference.Grant the minter role to our minter wallet. Here is an example script for granting the minter role to our Minting API.
Environment | Minter wallet address |
---|---|
Testnet | https://api.sandbox.immutable.com/v1/chains returns minter_address |
Mainnet | https://api.immutable.com/v1/chains returns minter_address |
- Ensure your application is configured around the Minting API rate limits detailed here.
SDK Usage
Minting API functionality can be accessed by using the Immutable SDK as well as calling the APIs directly. The code examples on this page refer to both approaches. When using the SDK approach, please ensure you have set up the SDK client correctly in your project as per these instructions on how to set up an Immutable SDK client.
Minting Functions by Collection
The Minting API is currently compatible with Immutable's ERC721 and ERC1155 preset contracts, providing flexibility for both non-fungible tokens (NFTs) and semi-fungible tokens (SFTs).
- ERC1155: safeMintBatch
- ERC721: mintBatch
- ERC721: mintBatchByQuantity
The Minting API utilizes the safeMintBatch()
function for ERC1155 collections. This function is generally more gas-efficient when minting multiple instances of the same asset (i.e., identical token_id
), as it requires less blockchain storage.
Compatible Preset Contract:
When using the Minting API with ERC1155 collections:
- The `token_id` must be specified
- The `amount` must be greater than 0
- Metadata can only be passed on the first mint for a new `token_id`. Attempting to pass metadata for an existing token will result in a 409 conflict error
The mintBatch()
function is used when a token_id
is specified in the API call. This function supports the minting of single or multiple assets from the same collection in a single request.
Compatible Preset Contracts:
This function allows studios to specify the token_id
at the time of minting. Though it is less gas-efficient compared to mintBatchByQuantity()
, it is useful for scenarios like asset migrations where maintaining consistent token_id
s is essential.
The mintBatchByQuantity()
function is used when a token_id
is not specified in the API call. Instead, the token_id
is system-generated sequentially. This method is more gas-efficient but does not allow for token_id
specification.
Compatible Preset Contract:
When using mintBatchByQuantity()
:
- A unique internal `reference_id` must be provided for each asset.
- The Blockchain Data API endpoint can be used to map the on-chain `token_id` to the off-chain `reference_id`.
Using the Minting API
Select on the tabs below for a step-by-step guide on how to mint with the different contract functions:
- ERC721 mintBatch(): With Metadata
- ERC721 mintBatch(): Without Metadata
- ERC721 mintBatchByQuantity(): With Metadata
- ERC721 mintBatchByQuantity(): Without Metadata
- ERC1155 safeMintBatch()
The mintBatch() function of Immutable's recommended ERC721 and Mint-By-ID preset contracts are used when token_id
is specified in the mint request.
The example below demonstrates including metadata with the mint request, which is the recommended method for optimizing system performance. By doing so, the Blockchain Data API doesn't need to crawl a game's baseURI/token_id
metadata directory, leading to an improved player experience.
However, game studios must ensure that the asset's metadata file is still placed in the baseURI/token_id
directory at the time of mint. This synchronization is essential for Immutable's Blockchain Data API, as some ecosystem partners may depend on the original metadata source file to publish an asset's metadata (e.g., Immutable Explorer).
Sequence of Actions for using ERC721 mintBatch() with Metadata
- Prepare the metadata for future assets in the specified format.
- Store the metadata in your
baseURI/token_id
directory. - Utilise the Minting API, specifying the required
token_id
in the API call, and include the metadata prepared in Step 1. Provide and usereference_id
to check the status of the mint request.
Example: Minting API with metadata in API call
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC721 collection | Body | Yes |
owner_address | The wallet address of the player who will own the minted NFT | Body | Yes |
token_id | An optional unique identifier within the collection for the NFT you are creating. If this is not added, we will create an ID for you. | Body | No |
reference_id | The internal content creator reference ID of the asset being minted. Each number must be unique for each collection's successful mints. Can be the same value as token_id . | Body | Yes |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallet's private key. | Header | Yes |
Run the following command to mint
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequestWithTokenIdAndMetadata(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string,
token_id: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
token_id,
metadata: {
name: "Brown Dog Green Car",
description: "This NFT is a Brown Dog in a Green Car",
image: "https://mt-test-2.s3.ap-southeast-2.amazonaws.com/BDGC.png",
external_url: null,
animation_url: null,
youtube_url: null,
attributes: [
{
trait_type: "Pet",
value: "Dog",
},
{
trait_type: "Pet Colour",
value: "Brown",
},
{
trait_type: "Vehicle",
value: "Car",
},
{
trait_type: "Vehicle Colour",
value: "Green",
},
],
},
},
{
owner_address,
reference_id,
token_id,
metadata: {
name: "Brown Dog Red Car",
description: "This NFT is a Brown Dog in a Red Car",
image: "https://mt-test-2.s3.ap-southeast-2.amazonaws.com/BDRC.png",
external_url: null,
animation_url: null,
youtube_url: null,
attributes: [
{
trait_type: "Pet",
value: "Dog",
},
{
trait_type: "Pet Colour",
value: "Brown",
},
{
trait_type: "Vehicle",
value: "Car",
},
{
trait_type: "Vehicle Colour",
value: "Red",
},
],
},
},
],
},
});
}
POST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
A successful request will receive a response like the one shown below:
{
"imx_mint_requests_limit": "2000",
"imx_mint_requests_limit_reset": "2024-02-13 07:20:00.778434 +0000 UTC",
"imx_mint_requests_retry_after": "59.98-seconds",
"imx_remaining_mint_requests": "1999"
}
The mintBatch() function of Immutable's recommended ERC721 and Mint-By-ID preset contracts are used when token_id
is specified in the mint request.
The example below demonstrates using the Minting API without including metadata in the mint request, which is not recommended. This approach requires the Blockchain Data API to crawl the metadata baseURI/token_id
directory, which can impact system performance.
If you choose to use this method, Immutable strongly advises that your metadata files are properly organized within the baseURI/token_id
directory structure. Failure to do so will necessitate additional metadata refresh calls after the minting process.
For more information on preparing metadata pre-mint, please refer to our documentation.
Sequence of actions for using ERC721 mintBatch() without metadata
- Prepare the future assets' metadata in the specified format
- Store the metadata in your
baseURI/token_id
directory - Utilise the Minting API, specifying the required
token_id
in the API call
Example: Minting API without metadata
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC721 collection | Body | Yes |
owner_address | The wallet address of the player who will own the minted NFT | Body | Yes |
token_id | An optional unique identifier within the collection for the NFT you are creating. If this is not added, we will create an ID for you. | Body | No |
reference_id | The internal content creator reference ID of the asset being minted. Each number must be unique for each collection's successful mints. Can be the same value as token_id . | Body | Yes |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
Run the following command to mint
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequestWithTokenId(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string,
token_id: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
token_id,
},
],
},
});
}
POST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
A successful request will receive a response like the one shown below:
{
"imx_mint_requests_limit": "2000",
"imx_mint_requests_limit_reset": "2024-02-13 07:20:00.778434 +0000 UTC",
"imx_mint_requests_retry_after": "59.98-seconds",
"imx_remaining_mint_requests": "1999"
}
The mintBatchByQuantity() function of Immutable's recommended ERC721 preset contract is used when token_id
is not specified in the mint request. reference_id
will be used to map a Minting API request to the system generated token_id
via the Blockchain Data API.
This method offers the most gas-efficient minting process.
The example below demonstrates including metadata with the mint request, which is the recommended method for optimizing system performance. By doing so, the Blockchain Data API doesn't need to crawl a game's baseURI/token_id
metadata directory, leading to an improved player experience.
However, game studios must ensure that the asset's metadata file is still placed in the baseURI/token_id
directory at the time of mint. This synchronization is essential for Immutable's Blockchain Data API, as some ecosystem partners may depend on the original metadata source file to publish an asset's metadata (e.g., Immutable Explorer).
If you do not prepare your metadata files within 10 seconds of receiving the token_id
, you may need to perform a metadata refresh on the assets to update ecosystem partners such as marketplaces. This can be rectified at any time by performing a metadata refresh.
For more information on preparing metadata pre-mint, please refer to our documentation.
Sequence of actions for using Minting API with metadata
- Mint the asset via the Minting API with the
reference_id
and corresponding metadata in the mint request. - Use webhooks or poll the
mint-requests
endpoint for updates on mint status and to establish thereference_id
->token_id
mapping. - Create a metadata file
../[baseURI]/[token_id]
and populate it with the metadata used in Step 1.
Example: Minting API with Metadata in the Request
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC721 collection | Body | Yes |
owner_address | The wallet address of the player who will own the minted NFT | Body | Yes |
reference_id | The internal content creator reference ID of the asset being minted. This internal ID is used to link the request to the system-generated token ID. Each number must be unique for each collection's successful mints | Body | Yes |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
- Run the following command to mint:
- SDK
- API
create-erc721-mint-quantity-request-with-metadata.tsView on GitHubimport { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequestWithMetadata(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
metadata: {
name: "Brown Dog Green Car",
description: "This NFT is a Brown Dog in a Green Car",
image: "https://mt-test-2.s3.ap-southeast-2.amazonaws.com/BDGC.png",
external_url: null,
animation_url: null,
youtube_url: null,
attributes: [
{
trait_type: "Pet",
value: "Dog",
},
{
trait_type: "Pet Colour",
value: "Brown",
},
{
trait_type: "Vehicle",
value: "Car",
},
{
trait_type: "Vehicle Colour",
value: "Green",
},
],
},
},
{
owner_address,
reference_id,
metadata: {
name: "Brown Dog Red Car",
description: "This NFT is a Brown Dog in a Red Car",
image: "https://mt-test-2.s3.ap-southeast-2.amazonaws.com/BDRC.png",
external_url: null,
animation_url: null,
youtube_url: null,
attributes: [
{
trait_type: "Pet",
value: "Dog",
},
{
trait_type: "Pet Colour",
value: "Brown",
},
{
trait_type: "Vehicle",
value: "Car",
},
{
trait_type: "Vehicle Colour",
value: "Red",
},
],
},
},
],
},
});
}📚API referencePOST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
A successful request will receive a response like the one shown below:
{
"imx_mint_requests_limit": "2000",
"imx_mint_requests_limit_reset": "2024-02-13 07:20:00.778434 +0000 UTC",
"imx_mint_requests_retry_after": "59.98-seconds",
"imx_remaining_mint_requests": "1999"
} - To verify the mint status and determine the `token_id` from the `reference_id`, follow [these steps](#get-minting-status-of-a-single-reference-id).
- Prepare a metadata file with a filename equal to the `token_id`. Save this file in the following location corresponding to the `baseURI` of your collection: `../[baseURI]/[token_id]`
The mintBatchByQuantity() function in Immutable's recommended ERC721 preset contract is used when token_id
is not specified in the mint request. Instead, the reference_id
is employed to map the Minting API request to the system-generated token_id
via the Blockchain Data API.
This method offers the most gas-efficient minting process.
The example below illustrates using the Minting API without including metadata in the mint request, which is not recommended.
If you choose this approach, once the token_id
is known, you will need to prepare the metadata files within the baseURI/token_id
directory structure. A metadata refresh will be required post-mint to update the Blockchain Data API and notify ecosystem partners of the newly minted asset's metadata.
For more information on preparing metadata pre-mint, please refer to our documentation.
Sequence of actions for using Minting API without metadata
- Mint the asset via the Minting API with the
reference_id
and no metadata. - Use webhooks or poll the
mint-requests
endpoint for updates on mint status and to establish thereference_id
->token_id
mapping. - Create a metadata file
../[baseURI]/[token_id]
and populate it with metadata used in Step 1 above. - Trigger a metadata refresh to update Immutable's Blockchain Data API.
Example: Minting API without metadata in request
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC721 collection | Body | Yes |
owner_address | The wallet address of the player who will own the minted NFT | Body | Yes |
reference_id | The internal content creator reference ID of the asset being minted. This internal ID is used to link the request to the system-generated token ID. Each number must be unique for each collection's successful mints | Body | Yes |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
Run the following command to mint
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequest(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
},
],
},
});
}
POST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
The Minting API uses the safeMintBatch()
function of Immutable's ERC1155 preset contract.
Compared to ERC721 it is possible to send multiple mint requests with the same token ID, either in one HTTP request or multiple requests. However, it is only possible to pass metadata the first time a token ID is used.
Immutable strongly recommends sending metadata to the API when creating a new token_id
. Additionally, ensure your metadata files are saved in your local directory in the baseURI/token_id
file structure. This practice ensures your assets are correctly represented on marketplaces and Immutable Explorer.
If you do not prepare your metadata files in your local directory in the baseURI/token_id
file structure, you may need to perform a metadata refresh post-mint to ensure all ecosystem partners, such as marketplaces, are updated with your asset's metadata.
For more information on preparing metadata pre-mint, please refer to our documentation.
Parameters to use Minting API for ERC1155 collections
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC1155 collection | Body | Yes |
owner_address | The wallet address of the player who will own the minted SFT | Body | Yes |
reference_id | The internal content creator reference ID of the asset being minted. This internal ID is used to link the request to the system-generated token ID. Each number must be unique for each collection's successful mints | Body | Yes |
token_id | A unique identifier within the collection for the SFT you are creating. | Body | Yes |
amount | How many tokens to mint for this token ID. | Body | Yes |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
Using ERC1155 safeMintBatch() to create a new SFT
The Minting API can be utilised to create an ERC1155 SFT by using the API the same way as ERC721 mintBatch() with an additional amount
field.
Run the following command to mint
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequestWithTokenIdAndMetadataAndAmount(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string,
token_id: string,
amount: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
token_id,
amount,
metadata: {
name: "Brown Dog Red Car",
description: "This SFT is a Brown Dog in a Red Car",
image: "https://mt-test-2.s3.ap-southeast-2.amazonaws.com/BDRC.png",
external_url: null,
animation_url: null,
youtube_url: null,
attributes: [
{
trait_type: "Pet",
value: "Dog",
},
{
trait_type: "Pet Colour",
value: "Brown",
},
{
trait_type: "Vehicle",
value: "Car",
},
{
trait_type: "Vehicle Colour",
value: "Red",
},
],
},
},
],
},
});
}
POST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
Using ERC1155 safeMintBatch() to mint to an existing SFT
Minting additional SFTs for a specific token ID looks almost the same as creating a new SFT. The main difference is that metadata cannot be passed anymore. If you need to change the metadata on an existing SFT please refer to our Metadata Refresh APIs. The minting API necessitates that your system distinguishes between creating a new Token ID and increasing the value of an existing Token ID. Metadata must be included in the initial request for creating the Token ID, while it should be omitted when adding quantities to existing Token IDs.
Providing metadata in an ERC1155 Minting API request for an existing SFT will result in a 409 conflict response.
Example: Minting API to mint additional SFTs
Run the following command to mint
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function createMintRequestWithTokenIdAndMetadataAndAmount(
chainName: string,
contractAddress: string,
owner_address: string,
reference_id: string,
token_id: string,
amount: string
): Promise<blockchainData.Types.CreateMintRequestResult> {
return await client.createMintRequest({
chainName,
contractAddress,
createMintRequestRequest: {
assets: [
{
owner_address,
reference_id,
token_id,
amount,
},
],
},
});
}
POST {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
Verifying the status of a Minting request
The mint-requests
webhook or polling endpoint can be utilised to check the status of a Minting API request.
For successful mints, the following data will be returned:
chain
reference_id
status
contract_address
owner_address
token_id
amount
activity_id
transaction_hash
created_at
updated_at
For unsuccessful mints, the following data will be returned:
chain
reference_id
status
error
contract_address
owner_address
created_at
updated_at
The status
field can have the following values:
- Succeeded: The mint was successful
- Pending: The mint request is waiting in a queue
- Fail: The mint was unsuccessful; see
error
for more details
These endpoints can be queried using the below methods.
- Minting Status: By Reference ID
- Minting Status: By Collection
To retrieve the status of a single reference_id
, execute the following command:
Parameter | Description | Location | Required |
---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes |
contract_address | The contract address of the ERC721 or ERC1155 collection | Body | Yes |
reference_id | The internal content creator reference ID of the asset being minted. This internal ID is used to link the request to the system-generated token ID. | Body | Yes |
chain_id | String representing the ID of the chain. A list of available chain IDs can be found here. | Response | Yes |
token_id | The system-generated token_id revealed once the asset has been minted | Response | No |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function getMintRequest(
chainName: string,
contractAddress: string,
referenceId: string
): Promise<blockchainData.Types.ListMintRequestsResult> {
return await client.getMintRequest({ chainName, contractAddress, referenceId });
}
GET {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests/{reference_id}
This will return the following results if the request is in a pending state.
{
"page": {
"next_cursor": null,
"previous_cursor": null
},
"result": [
{
"chain": {
"id": "CHAIN_ID",
"name": "CHAIN_NAME"
},
"collection_address": "CONTRACT_ADDRESS",
"created_at": "2024-02-12T05:35:35.743242Z",
"error": null,
"owner_address": "0x68209e7086032941a8Cb14352c2F43b086288791",
"reference_id": "REFERENCE_ID",
"status": "pending",
"token_id": null,
"activity_id": "null",
"transaction_hash": null,
"updated_at": "2024-02-12T05:35:35.743242Z"
},
]
}
The request will return the following request when it is successful, allowing you to map the REFERENCE_ID -> TOKEN_ID
{
"page": {
"next_cursor": null,
"previous_cursor": null
},
"result": [
{
"chain": {
"id": "CHAIN_ID",
"name": "CHAIN_NAME"
},
"collection_address": "0xe2e94d611d50370612e9721254807b7874093fb6",
"created_at": "2024-02-12T05:35:35.743242Z",
"error": null,
"owner_address": "0x68209e7086032941a8Cb14352c2F43b086288791",
"reference_id": "REFERENCE_ID",
"status": "succeeded",
"token_id": "TOKEN_ID",
"activity_id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"transaction_hash": "0x6890c450a43e6f3e90b311e2c0e80e1e6880cbc93ab977fc5357ac66cd255800",
"updated_at": "2024-02-12T05:35:35.743242Z"
},
]
}
Get minting status of all Minting API requests for a collection
The preferred method of receiving Minting API status updates is via the Mint Requests webhook event.
In the below scenarios we should you how to use the Blockchain Data API mint-request
endpoint to recieve updates.
To get the status of all reference IDs used for a collection, the following command can be run:
Parameter | Description | Location | Required | |
---|---|---|---|---|
chain_name | String representing the name of the chain. A list of available chains can be found here. | Body | Yes | |
contract_address | The contract address of the ERC721 or ERC1155 collection | Body | Yes | |
reference_id | The internal content creator reference ID of the asset being minted. This internal ID is used to link the request to the system-generated token ID. | Body | Yes | |
chain_id | String representing the ID of the chain. A list of available chain IDs can be found here. | Response | Yes | |
token_id | The system-generated token_id revealed once the asset has been minted | Response | No | |
x-immutable-api-key | Secret key for your environment obtained from your Hub account. Follow this guide to set yours up. This is not your wallets private key. | Header | Yes |
- SDK
- API
import { blockchainData } from "@imtbl/sdk";
import { client } from "../lib";
export async function listMintRequests(
chainName: string,
contractAddress: string,
): Promise<blockchainData.Types.ListMintRequestsResult> {
return await client.listMintRequests({ chainName, contractAddress });
}
GET {baseURL}/v1/chains/{chain_name}/collections/{contract_address}/nfts/mint-requests
The request will return the following results, allowing you to map the reference_id
-> token_id
for successful requests.
The most recent mint requests will be located as the top of the results.
{
"page": {
"next_cursor": null,
"previous_cursor": null
},
"result": [
{
"chain": {
"id": "CHAIN_ID",
"name": "CHAIN_NAME"
},
"collection_address": "CONTRACT_ADDRESS",
"created_at": "2024-02-12T05:35:35.743242Z",
"error": null,
"owner_address": "0x68209e7086032941a8Cb14352c2F43b086288791",
"reference_id": "REFERENCE_ID",
"status": "succeeded",
"token_id": "TOKEN_ID",
"activity_id": "4e28df8d-f65c-4c11-ba04-6a9dd80e1e6",
"transaction_hash": "0x6890c450a43e6f3e90b311e2c0e80e1e6880cbc93ab977fc5357ac66cd255800",
"updated_at": "2024-02-12T05:35:35.743242Z"
},
{
"chain": {
"id": "CHAIN_ID",
"name": "CHAIN_NAME"
},
"collection_address": "CONTRACT_ADDRESS",
"created_at": "2024-02-12T05:35:35.743242Z",
"error": null,
"owner_address": "0x68209e7086032941a8Cb14352c2F43b086288791",
"reference_id": "REFERENCE_ID",
"status": "succeeded",
"token_id": "TOKEN_ID",
"activity_id": "4e28df8d-f65c-4c11-ba04-6a9dd47b179b",
"transaction_hash": "0x6890c450a43e6f3e90b311e2c0e80e1e6880cbc93ab977fc5357ac66cd255800",
"updated_at": "2024-02-12T05:35:35.743242Z"
}
]
}
Error handling
The following tables details some of the errors that can occur when using the Minting API
- Request Errors
- Transaction Errors
The following errors can occur when making a Minting API call.
If these errors occur no event will be visible in Immutable's Blockchain Data API as the request would not have been accepted
Request validation errors
Cause | Example Error Message | Type | Code |
---|---|---|---|
>100 mints in a single request | request body has an error: doesn't match schema #/components/schemas/CreateMintRequestRequest: Error at \"/assets\": maximum number of items is 100 | VALIDATION_ERROR | 400 |
Duplicate reference_id in the request | duplicated reference id: 109 | VALIDATION_ERROR | 400 |
Duplicate token_id in the request | duplicated token id: 109 | VALIDATION_ERROR | 400 |
request reference_id already exists | there are already mint requests for reference_ids: 109 | VALIDATION_ERROR | 400 |
amount passed for ERC721 collection | amount is only relevant for ERC1155 collections. We do accept `amount="1" for convenience but all other values will return an error | VALIDATION_ERROR | 400 |
request with token_id already exists | there are already mint requests for token_ids: 109 | CONFLICT | 409 |
token_id already minted | token_ids already minted: 109 | CONFLICT | 409 |
Metadata for existing ERC1155 tokens | token_ids already minted: 109, no metadata allowed | CONFLICT | 409 |
Request authentication errors
Cause | Example Error Message | Type | Code |
---|---|---|---|
Incorrect API key | security requirements failed: API key is invalid | UNAUTHORISED_REQUEST | 401 |
Collection and API keys associated with different environments | API Key is not allowed to access this collection | AUTHENTICATION_ERROR | 403 |
Minting API not enabled for API key | API Key is not sponsored | AUTHENTICATION_ERROR | 403 |
Rate limit exceeded | Too many requests | TOO_MANY_REQUESTS_ERROR | 429 |
Minting API Idempotency
Networks can have issues and a client might not receive a response, even though the mint request was accepted successfully. To make sure we never process a mint request twice we don't accept the same reference_id
twice.
In case of network failure you can safely re-submit any requests that you aren't sure have been accepted. If the Minting API has already processed a reference_id
you will get a 409 Conflict
error with the following body:
{
code: "CONFLICT",
message: "there are already mint requests for reference_ids: 100",
details: {
id: "reference_id",
values: ["100"]
}
}
The following errors can occur after making a Minting API call.
These errors occur once a Blockchain Data API event has been triggered. They are visible from the mint request polling endpoint or the imtbl_zkevm_mint_request_updated webhook event.
Transaction errors
Cause | Example Error Message | Recommended Action |
---|---|---|
Minter role of collection not granted to Minting API | transaction failed to build: execution reverted: AccessControl: account 0x7ca12c6843adddbccdeb0efdf69a01028e274a70 is missing role 0x4d494e5445525f524f4c45000000000000000000000000000000000000000000 | Grant minter role to Minting API and try the request again. |
Minting API failed to submit transaction | Exceeded a maximum of 5 submissions | Submit request again |