On-Ramp tokens
The ONRAMP flow is a drop-in solution for web-based games and marketplaces that simplifies the process of purchasing tokens with fiat (e.g. USD) onto the Immutable zkEVM network.
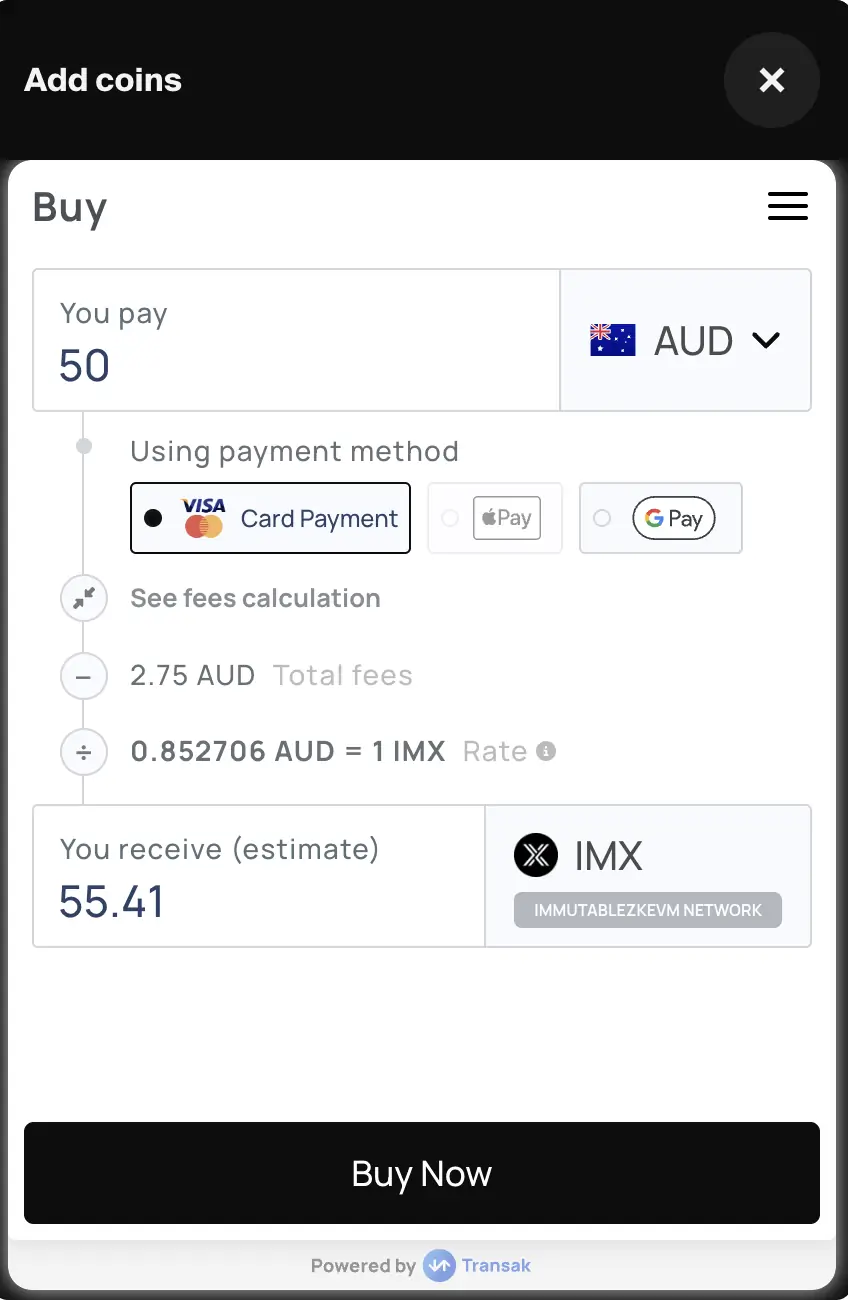
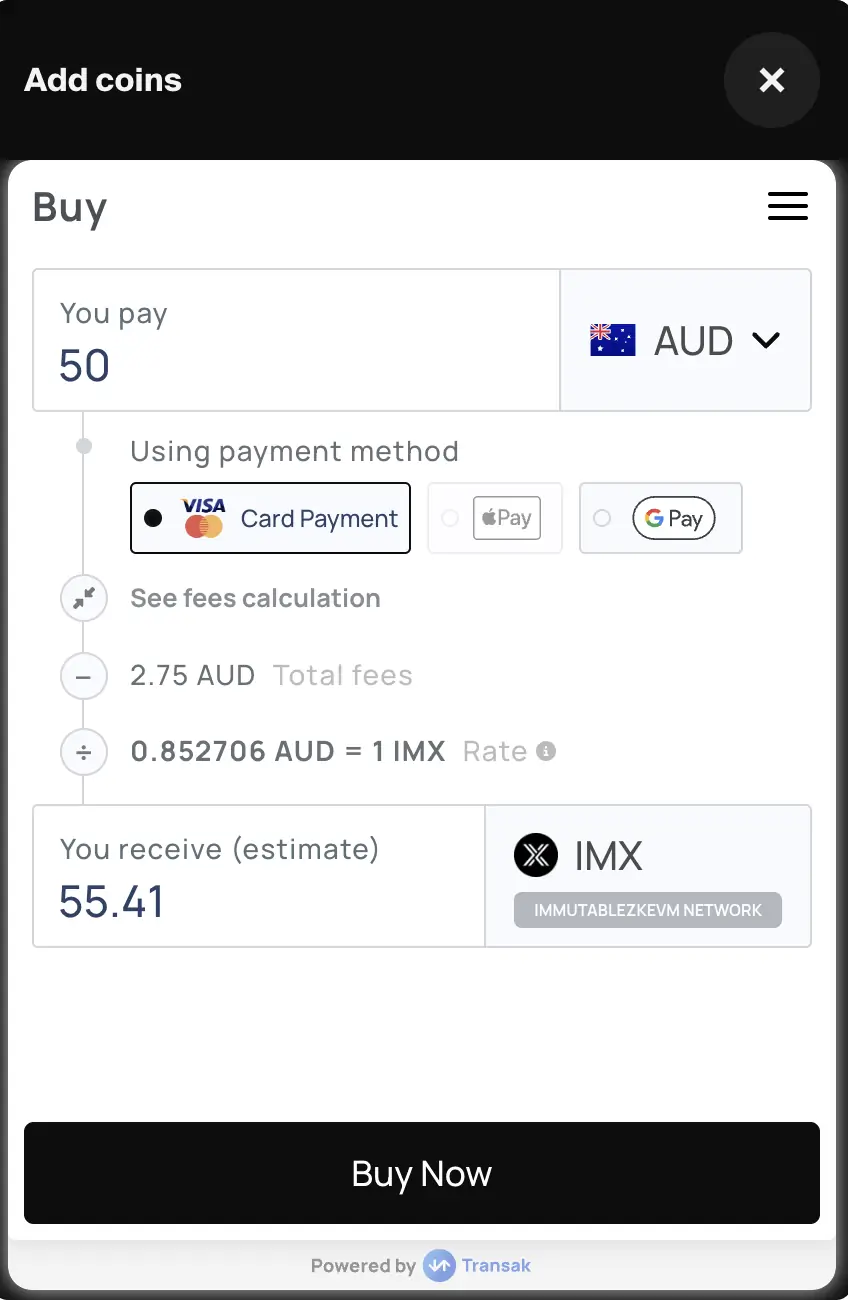
To request a new token is added, please follow Transak's On-ramp Token Listing Process.
Getting started
Once you have completed the widget setup, use the WidgetsFactory to create an on-ramp widget.
In order to mount the widget, call the mount()
function and pass in the id
attribute of the target element you wish to mount it to.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount an onramp flow, optionally pass any OnrampWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.ONRAMP,
});
})();
</script>
</body>
</html>
Parameters
The mount()
function can also take in parameters to be passed into the widget.
Parameters are treated as transient and will be reset after the widget is unmounted.
Property | Type | Description |
---|---|---|
flow | CommerceFlowType.ONRAMP | The flow type to be used. |
tokenAddress | string | The address of the ERC20 contract to onramp. |
amount | string | The ERC20 token amount to onramp. |
walletProviderName | WalletProviderName | Enum representing the names of different wallet providers i.e PASSPORT or METAMASK |
showBackButton | boolean | Whether to show a back button on the first screen, on click triggers REQUEST_GO_BACK event |
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: { theme: checkout.WidgetTheme.DARK },
});
// When calling the mount function,
// set flow to ONRAMP and pass in the parameters to use
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
// set amount and token to on-ramp
amount: '100',
tokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
// When calling the mount function,
// set flow to ONRAMP and pass in the parameters to use
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
// set amount and token to on-ramp
amount: '100',
tokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme will create the widget with that theme. If this is not passed the configuration will be set by default.
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
// When creating the widget, pass in the configuration
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: {
language: 'en',
theme: checkout.WidgetTheme.DARK,
// onramp flow configuration options
ONRAMP: {},
},
});
// Update the widget config by calling update()
// @ts-ignore
widget.update({
config: {
theme: checkout.WidgetTheme.LIGHT,
ONRAMP: {},
},
});
import { checkout } from '@imtbl/sdk';
// When creating the widget, pass in the configuration
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: {
language: 'en',
theme: checkout.WidgetTheme.DARK,
// onramp flow configuration options
ONRAMP: {},
},
});
// Update the widget config by calling update()
// @ts-ignore
widget.update({
config: {
theme: checkout.WidgetTheme.LIGHT,
ONRAMP: {},
},
});
Events
The Commerce Widget emit events events when critical actions have been taken by the user or key states have been reached.
Below is a table outlining the key events associated with a ONRAMP
flow.
Event Type | Description | Event Payload |
---|---|---|
CommerceEventType.CLOSE | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. | |
CommerceEventType.SUCCESS | The user has completed the flow successfully. | CommerceSuccessEvent |
CommerceEventType.FAILURE | There has been an error in the flow. | CommerceFailureEvent |
- React
- JavaScript
You can use the addListener()
function to tap into the events and provider handlers. Use the removeListener()
function to stop listening to that event.
import { checkout } from '@imtbl/sdk';
//@ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: { theme: checkout.WidgetTheme.DARK },
});
// Add event listeners for the ONRAMP flow
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
// narrow the event to a successful onramp event
if (payload.type === checkout.CommerceSuccessEventType.ONRAMP_SUCCESS) {
const { transactionHash } = payload.data;
console.log('successful onramp', transactionHash);
}
}
);
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
// narrow the event to a failed onramp event
if (payload.type === checkout.CommerceFailureEventType.ONRAMP_FAILED) {
const { reason, timestamp } = payload.data;
console.log('onramp failed', reason, timestamp);
}
}
);
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
console.log('widget closed');
});
// Remove event listeners for the ONRAMP flow
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.FAILURE);
widget.removeListener(checkout.CommerceEventType.CLOSE);
// Add event listeners for the ONRAMP flow
widget.addListener(ImmutableCheckout.CommerceEventType.SUCCESS, (payload) => {
// narrow the event to a successful onramp event
if (
payload.type === ImmutableCheckout.CommerceSuccessEventType.ONRAMP_SUCCESS
) {
const { transactionHash } = payload.data;
console.log('successful onramp', transactionHash);
}
});
widget.addListener(ImmutableCheckout.CommerceEventType.FAILURE, (payload) => {
// narrow the event to a failed onramp event
if (
payload.type === ImmutableCheckout.CommerceFailureEventType.ONRAMP_FAILED
) {
const { reason, timestamp } = payload.data;
console.log('onramp failed', reason, timestamp);
}
});
widget.addListener(ImmutableCheckout.CommerceEventType.CLOSE, () => {
widget.unmount();
console.log('widget closed');
});
// Remove event listeners for the ONRAMP flow
widget.removeListener(ImmutableCheckout.CommerceEventType.SUCCESS);
widget.removeListener(ImmutableCheckout.CommerceEventType.FAILURE);
widget.removeListener(ImmutableCheckout.CommerceEventType.CLOSE);
Sample code
This sample code gives you a good starting point for integrating the onramp widget into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [widget, setWidget] =
useState<checkout.Widget<typeof checkout.WidgetType.IMMUTABLE_COMMERCE>>();
// Initialise widget and mount an ONRAMP flow
useEffect(() => {
(async () => {
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
const checkoutWidget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
setWidget(checkoutWidget);
})();
}, []);
// mount widget and add event listeners
useEffect(() => {
if (!widget) return;
// On-ramp 100 USDC
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
amount: '100',
tokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
const { type, data } = payload;
// detect successful on-ramp
if (type === checkout.CommerceSuccessEventType.ONRAMP_SUCCESS) {
console.log('successful on-ramp', data.transactionHash);
}
}
);
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
const { type, data } = payload;
// detect when user fails to on-ramp tokens
if (type === checkout.CommerceFailureEventType.ONRAMP_FAILED) {
console.log('failed to on-ramp', data.reason);
}
}
);
// remove widget from view when closed
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
return () => {
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.CLOSE);
widget.removeListener(checkout.CommerceEventType.FAILURE);
};
}, [widget]);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
var web3Provider;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK, language: 'en' },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE,
{
config: {
WALLET: { showNetworkMenu: false },
},
}
);
// On-ramp 100 USDC
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.ONRAMP,
amount: '100',
tokenAddress: '0x3B2d8A1931736Fc321C24864BceEe981B11c3c57', // USDC
});
widget.addListener(
ImmutableCheckout.CommerceEventType.SUCCESS,
(payload) => {
const { type, data } = payload;
// detect successful on-ramp
if (
type === ImmutableCheckout.CommerceSuccessEventType.ONRAMP_SUCCESS
) {
console.log('successful on-ramp', data.transactionHash);
}
}
);
widget.addListener(
ImmutableCheckout.CommerceEventType.FAILURE,
(payload) => {
const { type, data } = payload;
// detect failed on-ramp
if (
type === ImmutableCheckout.CommerceFailureEventType.ONRAMP_FAILED
) {
console.log('failed to on-ramp', data.reason);
}
}
);
// remove widget from dom when closed
widget.addListener(ImmutableCheckout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
window.addEventListener('beforeunload', () => {
widget.removeListener(ImmutableCheckout.CommerceEventType.SUCCESS);
widget.removeListener(ImmutableCheckout.CommerceEventType.FAILURE);
widget.removeListener(ImmutableCheckout.CommerceEventType.CLOSE);
});
})();
</script>
</body>
</html>