Connect Wallets
The CONNECT flow simplifies the process of linking wallets and integrating into the Immutable zkEVM network.
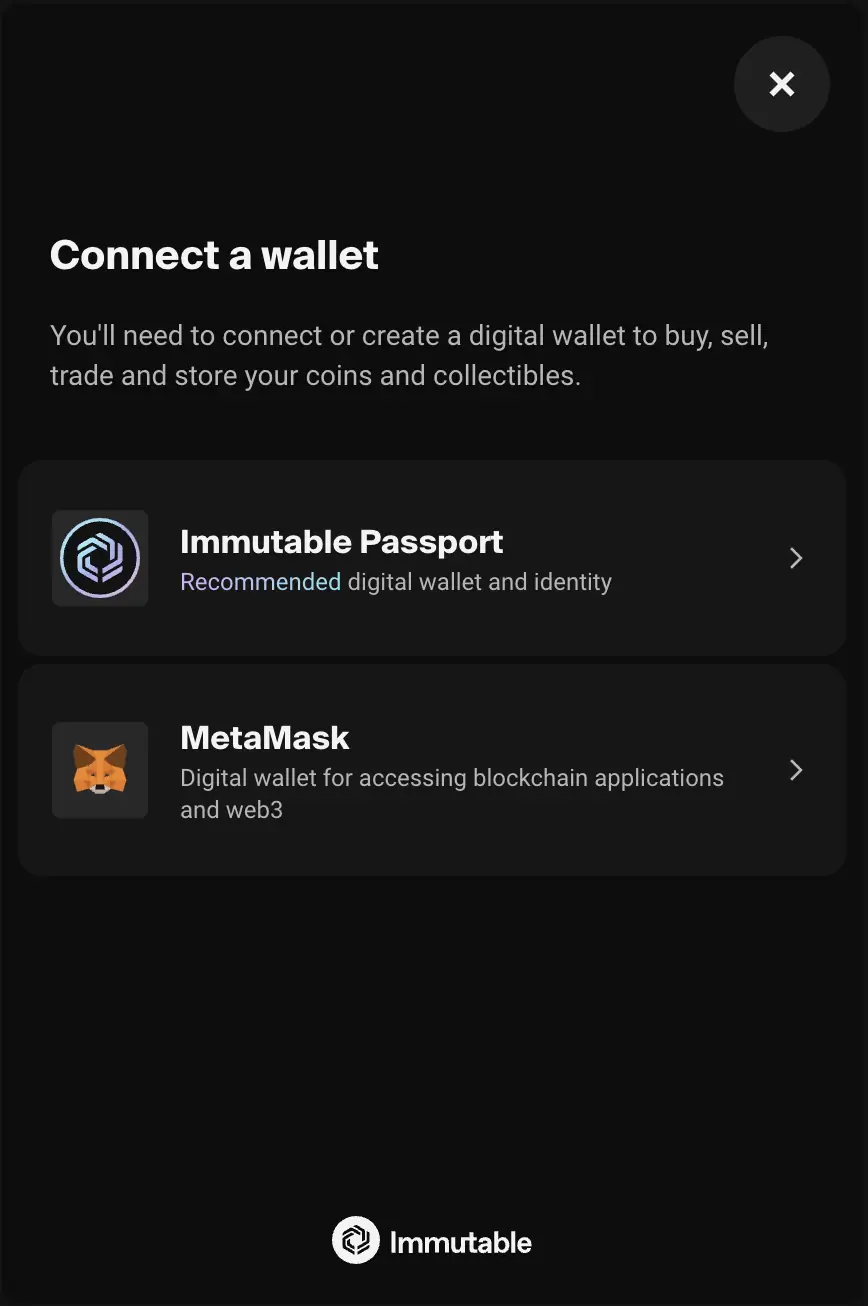
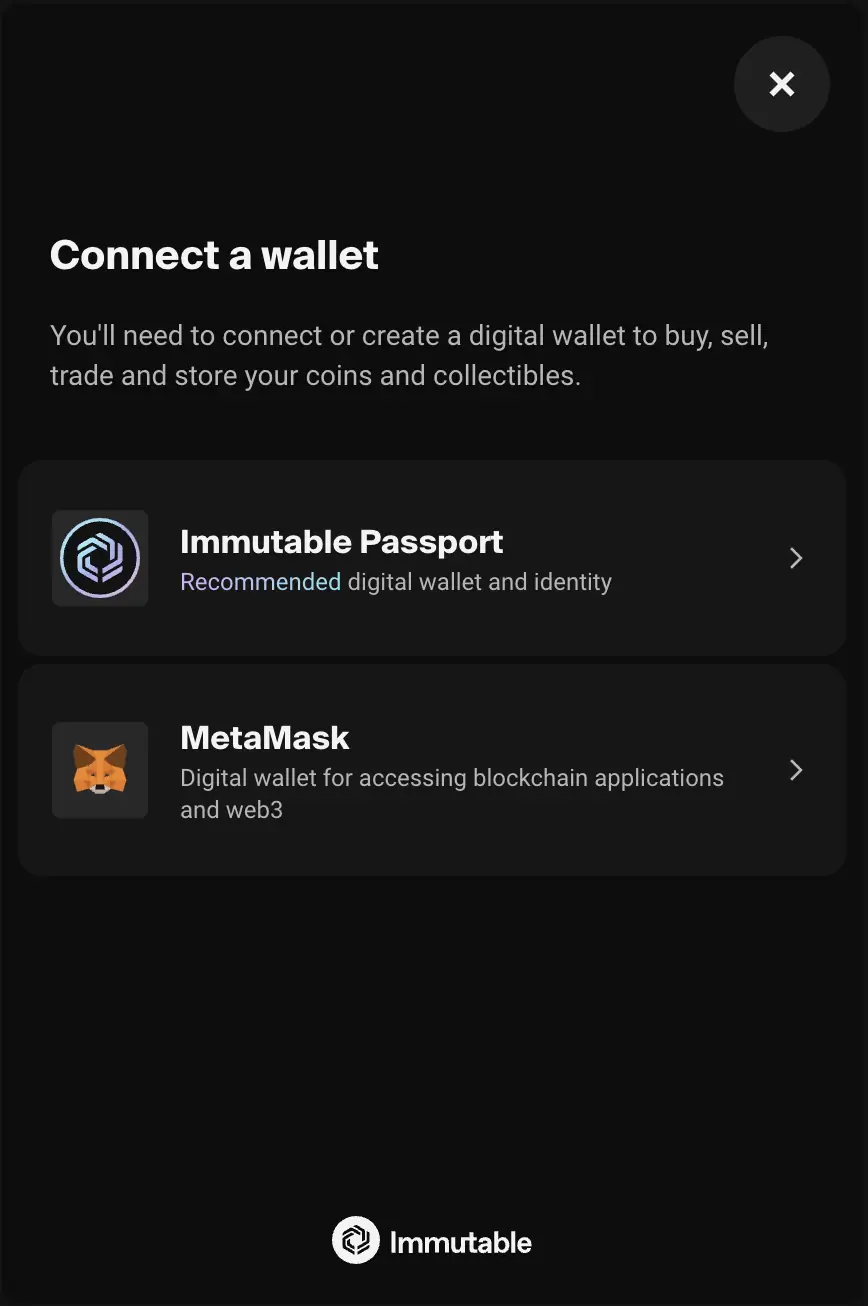
Getting started
Once you have completed the setup,
In order to initiate a flow call the mount()
function passing in the id
attribute of the target element you wish to mount the widget to,
and the parameters required by the CONNECT
flow.
- React
- JavaScript
import { useEffect } from 'react';
import { checkout } from '@imtbl/sdk';
// Create a Checkout SDK instance
const checkoutSDK = new checkout.Checkout();
export function App() {
// Initialise the Commerce Widget
useEffect(() => {
(async () => {
// Create a factory
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
// Create a widget
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
// Mount a connect flow, optionally pass any ConnectWidgetParams
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.CONNECT,
// Optionally, set connect behaviour
// blocklistWalletRdns: ['io.metamask'],
// targetWalletRdns: 'io.metamask',
// targetChainId: checkout.ChainId.IMTBL_ZKEVM_MAINNET,
});
})();
}, []);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="connect"></div>
<script>
// Initialize Checkout SDK
var checkout;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const widgets = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const connect = widgets.create(ImmutableCheckout.WidgetType.CONNECT);
connect.mount('connect');
})();
</script>
</body>
</html>
Parameters
The mount()
function will take in a CommerceWidgetConnectFlowParams object.
Parameters are treated as transient and will be reset after the widget is unmounted.
Property | Type | Description |
---|---|---|
flow | CommerceFlowType.CONNECT | The flow type to be used. |
targetChainId | ChainId | The target chain to connect to as part of the connection process (defaults to Immutable zkEVM / Immutable zkEVM Testnet) |
targetWalletRdns | string | WalletProviderRdns | The target wallet to establish a connection with |
blocklistWalletRdns | string[] | List of wallets rdns to exclude from the connect |
Configuration
When you first create the widget, you can pass an optional configuration object to set it up. For example, passing in the theme or language will create the widget with that theme or language. If these are not passed the configuration will be set by default. You will also have the option to provide the configuration for each of the supported flows.
Configuration will persist after the widget is unmounted. You can always update a widget's configuration later by calling the update()
method.
Property | Description |
---|---|
CommerceWidgetConfiguration | The configuration type to be used by the Commerce Widget. |
- React
- JavaScript
import { checkout } from '@imtbl/sdk';
// When creating the widget, pass in the configuration
// @ts-ignore
const widget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: {
language: 'en',
theme: checkout.WidgetTheme.DARK,
CONNECT: {}
},
});
// Update the widget config by calling update()
// @ts-ignore
widget.update({
config: {
language: 'ko',
theme: checkout.WidgetTheme.LIGHT,
},
});
// When creating the widget, pass in the configuration
const connect = widgets.create(ImmutableCheckout.WidgetType.CONNECT,
{ theme: ImmutableCheckout.WidgetTheme.DARK }
);
// Update the widget config by calling update()
connect.update({config: { theme: ImmutableCheckout.WidgetTheme.LIGHT }});
For more information on the configurations for the Commerce Widget flow, please review the Widget factory section in our Setup page.
Events
The Commerce Widget emit events events when critical actions have been taken by the user or key states have been reached.
Below is a table outlining the key events associated with a CONNECT
flow.
Event Type | Description | Event Payload |
---|---|---|
CommerceEventType.CLOSE | The user clicked the close button on the widget. This should usually be wired up to call the widget's unmount() function. | |
CommerceEventType.SUCCESS | The user has completed the flow successfully. | CommerceSuccessEvent |
CommerceEventType.FAILURE | There has been an error in the flow. | CommerceFailureEvent |
- React
- JavaScript
You can use the addListener()
function to subscribe to events and provide handlers. Use the removeListener()
function to remove the subscription to that event.
import { checkout } from '@imtbl/sdk';
//@ts-ignore
const widget = widgets.create(checkout.WidgetType.IMMUTABLE_COMMERCE, {
config: { theme: checkout.WidgetTheme.DARK },
});
// Add event listeners for the CONNECT flow
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
// narrow the event to a connect success event
if (payload.type === checkout.CommerceSuccessEventType.CONNECT_SUCCESS) {
const { provider, walletProviderInfo, walletProviderName } = payload.data as checkout.ConnectionSuccess;
console.log('connected', provider, walletProviderInfo, walletProviderName);
}
}
);
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
// narrow the event to a connect failure event
if (payload.type === checkout.CommerceFailureEventType.CONNECT_FAILED) {
const { reason } = payload.data;
console.log('connect failed', reason);
}
}
);
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
console.log('widget closed');
});
// Remove event listeners for the CONNECT flow
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.FAILURE);
widget.removeListener(checkout.CommerceEventType.CLOSE);
You can use the addListener()
function to tap into the events and provider handlers. Use the removeListener()
function to stop listening to that event.
// add event listeners for the connect widget
connect.addListener(ImmutableCheckout.ConnectEventType.SUCCESS, (data) => {
console.log("success", data);
});
connect.addListener(ImmutableCheckout.ConnectEventType.FAILURE, (data) => {
console.log('failure', data);
});
connect.addListener(ImmutableCheckout.ConnectEventType.CLOSE_WIDGET, (data) => {
console.log('close', data);
});
// remove event listeners for the connect widget
connect.removeListener(ImmutableCheckout.ConnectEventType.SUCCESS);
connect.removeListener(ImmutableCheckout.ConnectEventType.FAILURE);
connect.removeListener(ImmutableCheckout.ConnectEventType.CLOSE_WIDGET);
Sample code
This sample code gives you a good starting point for integrating the CONNECT flow into your application and listening to its events.
- React
- JavaScript
import { useEffect, useState } from 'react';
import { checkout } from '@imtbl/sdk';
// create Checkout SDK
const checkoutSDK = new checkout.Checkout();
export function App() {
const [widget, setWidget] =
useState<checkout.Widget<typeof checkout.WidgetType.IMMUTABLE_COMMERCE>>();
// Initialise widget and mount a CONNECT flow
useEffect(() => {
(async () => {
const factory = await checkoutSDK.widgets({
config: { theme: checkout.WidgetTheme.DARK, language: 'en' },
});
const checkoutWidget = factory.create(checkout.WidgetType.IMMUTABLE_COMMERCE);
setWidget(checkoutWidget);
})();
}, []);
// mount widget and add event listeners
useEffect(() => {
if (!widget) return;
widget.mount('mount-point', {
flow: checkout.CommerceFlowType.CONNECT,
});
widget.addListener(
checkout.CommerceEventType.SUCCESS,
(payload: checkout.CommerceSuccessEvent) => {
const { type, data } = payload;
// capture provider after user connects their wallet
if (type === checkout.CommerceSuccessEventType.CONNECT_SUCCESS) {
console.log('connected to ', (data as checkout.ConnectionSuccess).walletProviderName);
// setProvider(data.provider);
// optional, immediately close the widget
// widget.unmount();
}
}
);
// detect when user fails to connect
widget.addListener(
checkout.CommerceEventType.FAILURE,
(payload: checkout.CommerceFailureEvent) => {
const { type, data } = payload;
if (type === checkout.CommerceFailureEventType.CONNECT_FAILED) {
console.log('failed to connect', data.reason);
}
}
);
// remove widget from view when closed
widget.addListener(checkout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
return () => {
widget.removeListener(checkout.CommerceEventType.SUCCESS);
widget.removeListener(checkout.CommerceEventType.DISCONNECTED);
widget.removeListener(checkout.CommerceEventType.CLOSE);
};
}, [widget]);
return <div id="mount-point" />;
}
<html>
<head>
<!-- Load the SDK from jsdelivr -->
<script src="https://cdn.jsdelivr.net/npm/@imtbl/sdk/dist/browser/checkout/sdk.js"></script>
</head>
<body>
<div id="mount-point"></div>
<script>
// Initialize Checkout SDK
var checkout;
var web3Provider;
(async function () {
checkout = new ImmutableCheckout.Checkout();
const factory = await checkout.widgets({
config: { theme: ImmutableCheckout.WidgetTheme.DARK },
});
const widget = factory.create(
ImmutableCheckout.WidgetType.IMMUTABLE_COMMERCE,
{
theme: ImmutableCheckout.WidgetTheme.DARK,
}
);
widget.mount('mount-point', {
flow: ImmutableCheckout.CommerceFlowType.CONNECT,
});
widget.addListener(
ImmutableCheckout.CommerceEventType.SUCCESS,
(data) => {
// capture provider after user connects their wallet
if (
data.type ===
ImmutableCheckout.CommerceSuccessEventType.CONNECT_SUCCESS
) {
console.log('connected to', data.walletProviderName);
web3Provider = data.provider;
}
}
);
widget.addListener(
ImmutableCheckout.CommerceEventType.FAILURE,
(data) => {
// detect when user fails to connect
if (
data.type ===
ImmutableCheckout.CommerceFailureEventType.CONNECT_FAILED
) {
console.log('connect failed', data.reason);
}
}
);
// remove widget from dom when closed
widget.addListener(ImmutableCheckout.CommerceEventType.CLOSE, () => {
widget.unmount();
});
// clean up event listeners
window.addEventListener('beforeunload', () => {
widget.removeListener(ImmutableCheckout.CommerceEventType.SUCCESS);
widget.removeListener(ImmutableCheckout.CommerceEventType.FAILURE);
widget.removeListener(ImmutableCheckout.CommerceEventType.CLOSE);
});
})();
</script>
</body>
</html>